For loop in C
Learn via video course
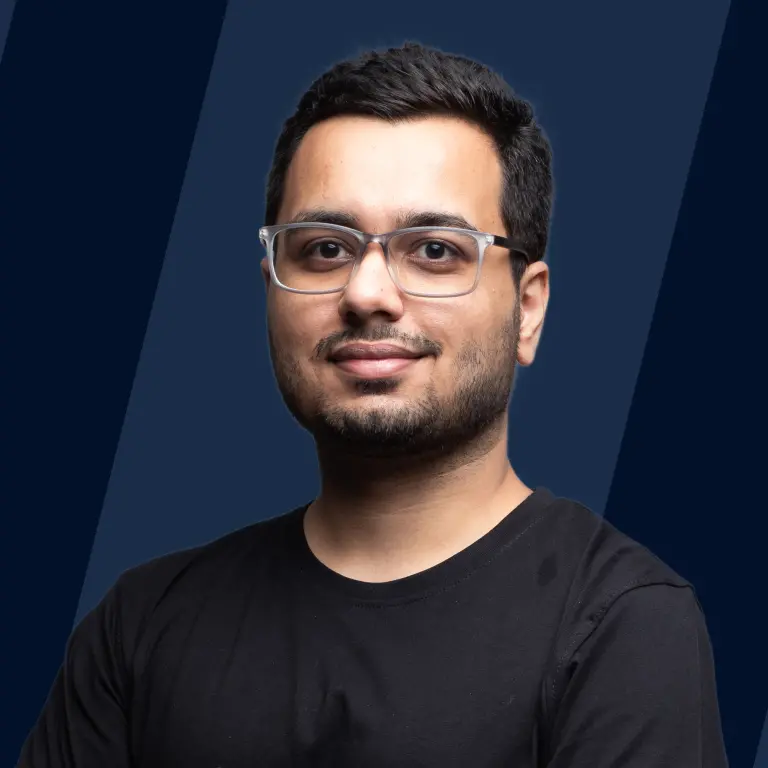
Overview
A for loop is a control structure that is used to run a block of instructions multiple times until a certain condition fails. It is used to traverse data structures such as arrays, strings, linked-lists and is commonly used in everyday programming.
Scope
In this article, we will be looking at:
- What is the for loop in C?
- For loop examples in C
- A few different ways to declare and run for loops in the C language.
- How it compares with the while loop?
Introduction to for loop in C
Imagine a company maintains an array salary that contains the salaries of its employees that they should receive every month. During the festive months of November-December, the company happens to decide to give each employee a bonus of 15% their salary. Hence, the company will have to create a new array updatedSalary that contains the salaries to be given to their employees that have their bonuses updated.
To do this, the tech team will use a for loop to first calculate the salary+bonus of each employee, and then update the new array with this sum.
How this occurs, is that a loop is run on the array salary where each element of the array is appended, the bonus is calculated using the formula (0.15 * salary), and then is added to the original salary. This sum is then appended into the new array updatedSalary where it is stored in the same position as the one in salary
We shall now look at the for loop syntax:
syntax of for loop C
The for loop syntax in c is as follows:
The initialization statement states the starting condition for the loop. It is run only once. As long as the semicolon appears, we aren’t required to put a statement here.
The condition statement is used to control the flow of execution of the loop based on some conditions. If this statement is not declared properly, it may lead to an infinite loop.
Finally, the update statement is used to update the value of loop control variables. This statement may even be left blank while running the loop.
Flowchart of for loop in C
Below is for loop flowchart in C.
for Loop Example
For the case of the company wanting to calculate their salary and bonus of the their employees that we have taken above:
OUTPUT:
Forms of for loop
Aside from the above syntax, there are many other ways we can run for loops. Some don't require an initialization, some don't require an updation, and some don't require both! Here are a few ways to run for loops:
A) NO INITIALIZATION:
Initialization can be skipped as shown below:
B) NO UPDATION:
We can run a for loop without needing an updation in the following way:
C) NO INITIALIZATION AND UPDATE STATEMENT:
We can avoid both initialization and update statements too!
If we notice carefully, we can see that this syntax is similar to the while loop. Readers can take note that using a while loop is a better choice than running a for loop with this syntax.
Comparision between for and while loop
The for loop and the while loop are structurally similar to each other, as both need to have the test condition declared before the loop and the program checks the test condition(s) each time before running the loop once again. While they look like they're doing the same thing, they have completely different use cases. The execution of both loops depends on the test expression.
The most fundamental difference between the two is that a for loop runs a preset number of times, whereas a while loop runs until a condition is met. This means that we may use for loops if we know how many times to iterate, and a while loop when we don't.
In general, use a while loop if you want the loop to break depending on something other than the number of times it runs.
Conclusion:
- For loops in c are used to iterate over a sequence.
- It iterates a preset number of times and stops as soon as conditions are met.
- In the absence of a condition, the loop will iterate indefinitely until it encounters the break command.
- Loops are thus a collection of instructions that must be used in a particular sequence.
- If the loop's structure is wrong, the programming will display a syntax error.
- Loops run to produce a result or to satisfy a condition or set of requirements.
- They are one of the most fundamental components in programming languages.