Uses of C++
Learn via video course
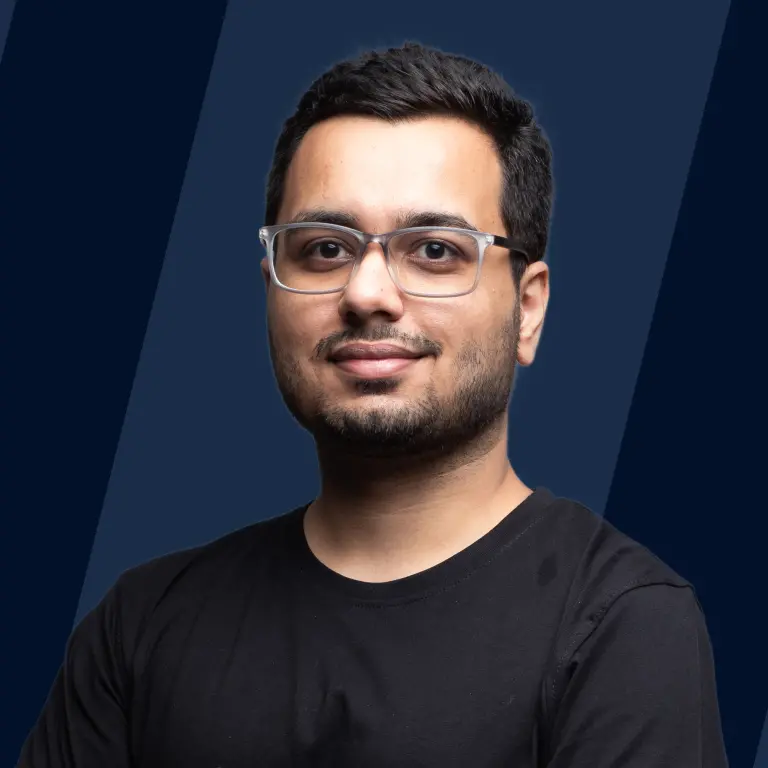
Overview
C++ is a general-purpose object-oriented programming language. C++ is faster and more reliable when compared to other high-level languages like Java and Python. For this reason, even today, C++ has a range of uses, from creating modern applications to designing database management systems (DBMS) to writing compilers for other languages. C++ also has enormous job opportunities as firms like Google, Amazon, Flipkart, IBM etc., hire C++ developers.
Scope of the Article
- This article begins with several benefits that C++ offers over other languages and gradually covers real-world applications built with C++ and uses of C++.
- The article also covers some popular repositories for open-source contributions in C++.
- In the end, we have discussed several job opportunities for a C++ developer with some valuable tips to learn and master C++.
What Makes C++ Unique?
C++ is a general-purpose, object-oriented programming language. C++ has become the language of choice for building extensive software infrastructure that needs to run on limited resources. Also, C++ programs can be used to interact with the computers harware, which a programmer can use efficiently to run the program in an environment with limited hardware space or energy available to power the device.
C++ applications run quickly and reliably on various devices, making it the preferred choice for writing the base layer of many critical applications. This is because C++ is a good choice for writing and building software that is fast, reliable in critical tasks, and efficient at managing system resources.
What Makes C++ Fast and Reliable?
C++ don't have extra overhead which degrades the performance of the application like garbage collection, dynamic typing, cheking array index bounds etc. Not adding such features make C++ process informations faster compared to many other languages but in the same time it means programmers need to be able to allocate and free memory to prevent memory leaks in the application and deal with static typed variables. Also, C++ gives programmers a lot of control over memory and has support for pointer.
Benefits of C++
There are lot of benefits and uses of C++. Here are some of the essential features of the language
- Speed: C++ is a powerful language that has tight data structures that means variables can store data of a particular type mentioned in the variable declaration. Additionally, C++ skips check like memory leaks and bound checking that prevents time to check these overheads making C++ a fast language.
- Efficiency in writing code: C++ has a standard template library (STL) that provides efficient algorithms and data structures that can be used to save programming time and effort that would otherwise be extended writing that code.
- Freedom to choose programming styles: C++ supports several different styles of writing code, such as C-style programming, Object-oriented programming etc. The programmer can choose a style that fits his use case making it useful for wide variety of cases.
- Object Oriented Programming language: C++ provides an ability to solve complex problems intuitively by creating custom objects. Also, C++ is partially an object-oriented programming language and not purely an OOPs language as prgrammers still can use it without using any of OOPs concept as the main() function (starting point) in C++ is not inside a class. Still, programmers can use OOPs concepts like data abstraction, encapsultaion and polymorphism and advanatages that comes along with OOPs to code.
- Catch errors before execution: C++ is a statically typed language, which means that variables can only hold a value of a particular data type that needs to be defined at the time of variable declaration. The benefit of C++ being a statically typed language is that the compiler can catch errors before executing the program, saving debugging times.
Advanced Concepts of C++ Language
File Handling
Storing data inside variables are temporary. Files are used to retain a large amount of data permanently. C++ allow users to access and create files. To perform such operations, C++ has a header file <fstream> that must be included.
As the image suggest file handling in C++ is mainly dealt by using three classes available in fstream headerfile.
- ofstream: Stream class used to write on files.
- ifstream: Stream class to write from file.
- fstream: It is stream class to both read and write in files.
The first step, when reading or write in file is opening the file. We can open file by using open() method by passing the file name as an argument.
Writing to a File
To write information in a file from C++ we need to use stream insertion operator (<<) just as we use this operator to output information on the screen and the only difference is we use ifstream or fstream object instead of the cin object.
Reading from a File
We can read information stored in the file using stream extraction operator (>>) just as we use the operator to input information from the keyboard with only difference is that we use ifstream or fstream object instead of the cin object.
Let us see an example code where we are trying to open a file by taking name of the file as an input from the user within a C++ program and write "Hello World" inside the file if the file already exists in the computer otherwise show an error message.
Enumerated Constants
There are several types of data like days of the week that are not inherently numeric. We can refer such data using symbolic constants that will symbolically represent our information.
For example,
Here, we are representing day of the week as in integer value so, monday is represented with an interger 0, tuesday as 1, and so on.
C++ has a convenient way to define such data using a new data type: the C++ enumeration statement.
That creates a new variable type with legal values Mon, Tue, Wed, Thru, Fri, Sat, and Sun. The compiler by default adds the value of first constant as 0, the value of second constant as 1, and so on. But these integer values are not used to access constant and we use the constant themselves to access the constants in C++.
To declare a variable day of type Days and set its value, we use syntax.
Lambda Expressions
C++ 11 and above has the support of lambda expressions that allow us to write inline functions that are not going to be reused or are not worth naming.
A lambda expression can have more power as it can capture external variables from enclosing scope of lambda expression using three ways:
- Capture by reference
- Capture by value
- Capture by both (mixed)
Syntax to write lambda expressions is
Capture clause can help to access extenal variables from expressions internal scope in three ways:
- Capture by reference [&]: Capture all external variables by their reference.
- Capture by value [=]: Capture all the external variables by their values.
- Capture by both [a, &b]: Capture a by value and capture b by its reference.
A lambda with empty capture clause [] can only access variables that are local to it.
We don't need to explicitly mention return type as the compiler itself evaluates the return type of the lambda expression but we might have to declare the -> return_type part in case of complex cases as in conditional statements, where compiler can not decide the return type correctly.
Let us see an example, where we are creating a custom lambda expression which is passed to C++ sort() function to sort array of numbers with the logic expressed in the lambda expression (decreasing order in this case).
Output
Here, we are sorting the nums array using the sort() function, but instead of defining a separate function and passing it to the sort() function, we are simply creating an inline lambda expression that is doing the same task. Notice we are not giving any particular name to our comparison function.
Contributing to Open-Source Projects
Open-source is generally referred to as open-source software (OSS). An OSS is the one that is available for free on the internet to use, modify and test. Users can add or remove a software patch according to their requirements. Contributing to open-source can improve your knowledge and skills. It also helps become a part of the significant community learning many social and technical skills in the journey. Adding cherry on a cake, your contributions will benefit millions of users using the software you contributed.
Some of the popular open-source platforms include
- GitHub
- Up For Grabs
- First Contributions
Be it tech giants like Adobe or trading companies like Bloomberg trading or popular games, they all have a common link between them that is use of C++ in there code base. Because of addition of OOPs concept in C++, it allowed programmers to use C++ for development of Web and Web-based applications which was previously very difficult with C. Because of the speed of C++, it is also used as a wrapper in many of the python libraries. Companies and several good projects build with C++ is avaible as open-source projects where developers can contribute. Contributing in open-source project based on C++ will help get a glism of how production ready codes are written with C++.
Popular C++ Repos
Many of the popular open-source software uses C++ in its code. There are several good projects built using C++ that you can contribute to. Some of them are as follows.
- Electron: It is an open-source project used to build cross-platform desktop apps with JavaScript, HTML, and CSS. the project has very clean and detailed documentation with about 1.5k open issues. The project has 101k stars, which shows that many people are interested in and following the project.
- openCV: openCV is an abbreviation of Open Source Computer Vision Library. It provides a real-time optimized Computer Vision library, tools, and hardware. It is a programming library having functions mainly aimed at real-time computer vision.
There are several other popular open-source projects built with C++. You can explore more of them from here.
Beginner-friendly C++ Repositories
- GoogleTest: GoogleTest is a C++ testing framework managed by google. In the repo's readme, you will find all the necessary information about this C++ project. You will find the list of several platforms where this C++ testing framework can be used in the readme file. Names of other open-source projects that are closely related to this repo are also mentioned in the project's readme. There are currently several open issues in the repository that you can choose to contribute. The repository has over a thousand stars showing the interest of several people in the project.
- RapidJSON: This project is Tencent's contribution to open-source, a fast JSON parser/generator for C++. The framework is very fast, which is why the project has rapid in its name. This project is self-contained and header-only. For this reason, you will not need any external library to run the project. It also is very memory efficient, and JSON exactly takes 16 bytes. The readme is also very well documented, and the repo also has several open issues making it a tremendous chance for contribution.
- ClickHouse: It is an open-source database management system that allows the generation of data reports in real-time when the data comes. The repo has a clean readme with all the details that the repo offers. They have a YouTube channel that can be used to clear some doubts you might have about this repository. The repo has significant issues to which you can contribute. The repo has thousands of stars, meaning many users are interested in and are working on this project.
Real-World Applications of C++
Let us see some of the real-world uses of C++.
Game Development
C++ language can have finer control over actual computer hardware, which is required in high-performance games and gaming engines. Also, its low-level programming abilities offers a high degree of flexibility that simply is not present in other higher-level languages. Because C++ allows ability to manipulate hardware and control memory management to optimize performance of the application it is good choice to built high performance games. For this reason, most of the game engines are written in C++ like Blender, GamePlayer3D, 4A Engine etc.
GUI based Applications
We can use C++ to develop most modern GUI applications. For example, many of the applications from Adobe, including Photoshop, Illustrator etc., are developed using C++. This is because software like Photoshop deals with a lot of data, large files, tons of layers and a lot of mathematics. To deal with such an amount of data top real-performance is top priority which C++ can deliver.
Database
C++ helps manage data by using its several features like speed, reliability, classes, functions, and file handling. Most major DBMS applications like Oracle, MySQL, Postgres and MongoDB are developed in C++.
Operating Systems
Operating systems need to be fast and efficient at handling computer resources, and because C++ is a high-speed programming language with several system-level functions, that makes it an ideal choice for writing OS. Microsoft Windows, Mac OS X, and even mobile OS like iOS was developed using C++.
Web Browsers
Most of the popular web browsers like Internet Explorer, Google Chrome, Mozilla Firefox, Safari etc., are powered by C++. C++ mainly handles the back-end services responsible for retrieving information from databases and converting code to interactive web pages this is because we want to quickly process the data and convert them to web pages to give users an immersive experience without any noticeable lags.
Advanced Computations and Graphics
C++ is used in developing software requiring high-performance like image processing, real-time physics simulation that need to process lots of data and performs mathematical operations in real-time with high-performance. For example, Maya 3D is developed using C++ and is used for animation and 3D graphics.
Banking Applications
C++ aids in concurrency, it becomes a good choice for banking applications that require multithreading, concurrency, and high performance. For example, Infosys Finacle is a popular core banking application that is written in C++ as a backend programming language.
Embedded Systems
C++ is closer to hardware and provides a range of low-level function calls compared to other high-level languages. It is used in various embedded systems like medical equipment and smart-wearables.
Enterprise Software
C++ is used in several enterprise software and advanced applications like radar processing and flight simulator mainly because of it's high-performance and memory management capabilities. C++ is good choice when it comes to process a lot of data in real-time.
Compiler
Various high-level programming languages have their compilers written in either C or C++. This is because both these languages are low-level languages and are much closer to hardware, and they are able to program, manage and manipulate the hardware resources efficiently. Learn more about online C++ compiler.
Cloud/Distributed Systems
Cloud storage systems work very close to the hardware, and for this, C++ becomes the language of choice for such solutions. Also, C++ provides multithreading support that is used to build concurrent applications along with load tolerance to the hardware system.
Job opportunities in C++
C++ is a comparatively more complex language to learn, but the range of applications that can be developed using C++ is astonishing. C++ is used in a range of applications and fields like finance, android, game and application development, virtual reality and many more for development roles. C++ also has a massive role in HFT (High Frequency Trading) firms as a quantitaive researcher.
Top-notch companies like Google, Amazon, Flipkart, IBM, Infosys, Tower Research, Sun Microsystems etc., hire C++ professionals.
How to Build a Career in C++
C++ is not an easy language to learn and master, especially for someone beginning their programming journey. Still, the range of applications and opportunities that C++ offers is enormous, and for this reason, the question we instead need to ask is How much C++ do we need to know to get a job in the field?. There is no correct answer to the question because there's always more to learn. It doesn't matter if someone has one month or ten years of experience. The more experience and skill one has with C++, the more likely one will land a respectable job.
There are different job roles that will help you build a career in C++ domains. A few of them are,
- Software developer
- Quality Analyst
- Backend Developer
- Database Developer
- Game Programmer
- C++ Analyst
- Embedded Engineer
C++ developers are in demand, and they enjoy high paying jobs in the industry. The average C++ developer salary in India is ~7.4 Lacs. However, it depends on an individual's skill set, qualifications and expertise.
Useful Tips and Other Things to Keep in Mind
Here are some valuable tips and things to keep in mind as you start your journey with C++
-
C++ takes time to master and learn compared to other high-level programming languages requiring a long term commitment and devotion of your time and energy to learn it. Depending on your commitment and previous experiences, the time to learn C++ will vary, but in any situation, you shouldn't expect to master C++ in a few days.
-
It can be overwhelming to learn C++ basics initially because there is a lot to go through. As such, take time to understand the fundamentals of the language and seek support from peers who have experience with the language.
-
Practice is the key because the more you will practice and take the time to apply what you learned, the more it will help you understand the concepts better.
-
Try to find errors and broken code and fix them because when you try to fix an issue or go through someone else's code, you are forcing yourself to tap into different areas that you have learned.
-
Join communities and attend/watch conferences. Many communities and platforms can help you with your C++ learning journey, like HackerRank, Codechef, Stackoverflow, Codeforces, and GeeksForGeeks.
Conclusion
- C++ is a general-purpose, object-oriented programming language that is very efficient and fast. C++ finds its use even today in the development of several modern applications.
- C++ is used to develop several modern GUI-based applications in cloud/distributed systems, web browsers, etc.
- C++ has wide range of applications and is used in various domains of software engineering that is from building high performance gaming engines like Blender to GUI based aplications like Adobe photoshop and in building critical systems like banking and in high frequency trading firms (like Quadeye and tower research) where speed and efficiency are of prime performance.
- C++ is a little harder to learn but has vast job opportunities as most popular firms like Google and Microsoft use C++ in their applications and hire people for roles related to C++.