Vector in C++
Learn via video course
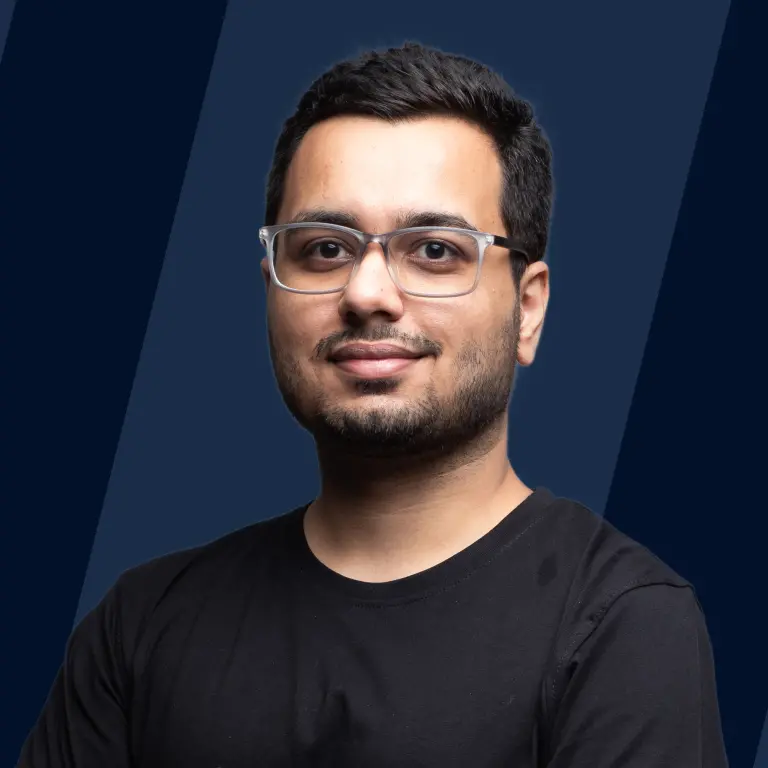
Overview
Vectors are containers representing arrays used to store elements of the same data type but the size of a vector grows and shrinks dynamically, that is, it can change its size during runtime. The elements of a vector are placed in contiguous memory locations so that they can be accessed and traversed using iterators.
Scope
- This article covers the concept of vectors in C++.
- It covers the difference between a vector and an array in C++.
- It covers STL library functions of Vector in C++.
Need for Vectors
Suppose you have to collect details of multiple employees in an organization. How would you do so?
You could create individual objects if employees are less in number. Also, a practical approach would be to create a linear data structure like an array or linked list.
But it's inappropriate to use these data structures here because of the following reasons:
- We are unaware of the number of inputs. Practically it is always unknown to us, how many inputs we will be getting.
- A linked list would require a linear traversal for accessing an element which makes
So are we left with any alternative that serves all these purposes in the least time?
Yes, vectors!
Introduction to Vector in C++
Vectors are one of the containers offered to us by the STL(Standard Template Library) in C++. It stores a collection of similar-type objects in a variable-sized array.
Note: C++ provides us with objects that store a collection of elements (i.e. other objects), referred to by the term ‘containers’.
What is a Vector in C++
Vector is a linear data structure that stores similar type objects. It is a template class in C++ STL(standard template library). A vector is implemented as an array which is free to add elements even beyond its predefined size. Basically, this array is controlled by the vector class and as soon as it goes out of bounds, the copy constructor copies it to another array of a bigger size. This bigger array is later used as our main internal array. Since internally copy is being created which takes time ao it is recommended to give size if known while initializing it.
We are provided the vector template by these header files –
Since both files provide us with the vector class for implementation on their own. Hence we need to include only one of the above header files to use vectors.
How to create vectors in c++?
We can create vectors in our programs using multiple techniques. It can be created either through
- declaration
- declaration with specific size or ( here elements are initialised by 0 as default value)
- declaration with specific size and initialisation with a specific value
Syntax:
In the case of multi-dimensional vectors. We consider a multidimensional vector as a vector of vectors.
This helps us to declare variable-sized vectors within the vectors which enables us to have memory efficiency. The image below shows how the 2D vector has variable sizes for its rows which saves us memory.
Code:
Difference Between Vector and Array
Insertion and Deletion
Array | Vector |
---|---|
After deletion, the user needs to do shifting of elements explicitly in the program. | The vector itself shifts all the elements internally. |
We cannot insert elements more than the size of the array. | We can insert elements more than the size of the vector using push_back() |
Array is static. | Vector is dynamic. |
Vector Insertion and Deletion Functions
Function | Description |
---|---|
vector::push_back(element); | It inserts the element at the end of the vector |
vector::pop_back(); | It removes the last element of the vector |
vector::insert(iterator, element); | It inserts the element at the position specified by the iterator passed to it. |
vector::clear(); | It removes all the values from the vector |
The easy insertion at the end of the vector with push_back will help us to add any number of values to our vector irrespective of the size it was declared with.
An Array would throw a segmentation error if we insert more elements than its size. Also, declaring an array with a large size may lead to memory wastage.
Code:
A walkthrough of how our vector is getting updated at each step
At the start | 10 | 20 | 30 | 40 | 50 | ||
---|---|---|---|---|---|---|---|
After push_back() | 10 | 20 | 30 | 40 | 50 | 60 | |
After insert() | 10 | 20 | 100 | 30 | 40 | 50 | 60 |
After pop_back() | 10 | 20 | 100 | 30 | 40 | 50 | |
After swap() | 2 | 4 | 6 | 8 | |||
After clear |
Copying
Array | Vector |
---|---|
We can copy one array to another only by iterating over the whole array and copying values to the other array of the same data type. | There are different ways to copy one vector to another vector::assign(); |
int a[5] = {1,2,3,4,5}; int b[5]; for (int i = 0; i < 5; i++) b[i] = a[i]; | Assignment Operator (=) Using Constructor vector::copy(); |
Code:
Size
Array | Vector |
---|---|
Arrays need to be necessarily provided with a size when they are declared | Vectors do not need to be provided with a size when they are declared as they are dynamic. |
Number of elements is given by sizeof(array_name)/sizeof(array_data_type); | No of elements is given by vector::size(); |
We cannot resize the array once declared. | We can resize the vector using the member function even after initialisation using vector::resize(new_size_of_vector); |
Code:
In a vector, the size() will help us to know the number of elements stored in it without traversal.
Also, we can save our storage space by resizing our original vector to a smaller size whenever required.
Iterators and traversals
Array | Vector |
---|---|
Array does not offer us any iterators so we have to depend on loops for traversal. | Vectors offer iterators which enable us to perform different types of traversals. |
Elements are accessed using [] operator | Elements are accessed using * operator |
code for traversing array
code for traversing Vector
Iterators offered by vectors
Function | Description |
---|---|
vector::begin() | It returns an iterator pointing to the first element of the vector. |
vector::end() | It returns an iterator pointing to the element next to the end element |
vector::rbegin(); | It returns an iterator pointing to the last element of the vector(first in the reversed vector). |
vector::rend(); | It returns an iterator pointing to the first element of the vector(last in the reversed vector). |
vector::cbegin() | It is similar to vector::begin(), but it doesn’t have the ability to modify elements. |
vector::cend() | It is similar to vector::end() but it doesn’t have the ability to modify elements. |
Code:
Modifiers
Array | Vector |
---|---|
We can update any element in the array by using its index and subscript operator [] | We can update an element in a vector just like an array by using its index and subscript operator []. We can also do so using iterators as discussed and also using vector::at(); function. |
int b[5] = {11,22,33,44,55}; b[3] = 0; //b = {11, 22, 33, 0, 55} | vector a.at(2) = 0; a[4] = -1; //a = {10, 20, 0, 40, -1, 6} |
Vector Functions in C++
This is a brief compilation of all the widely used vector functions.
Initialisation
Function | Description |
---|---|
vector::copy(start_iterator, end_iterator, back_inserter(vector)); | Copies a complete vector or the range of elements specified into another vector |
vector::assign(start_iterator, end_iterator); | Assigns all elements of vector with a specific value |
Insertion and Deletion
Function | Description |
---|---|
vector::push_back(); | Adds element to end of vector |
vector::pop_back(); | Removes element from end of vector |
vector::insert(iterator,value); | Adds an element at a particular index specified. |
vector::clear(); | Removes all elements from the vector a makes its size 0. |
vector::swap(vector); | Swaps contents of one vector with another vector of the same data type. |
vector::erase() | Removes elements from specific positions or ranges |
Size
Function | Description |
---|---|
vector::size(); | Returns the no of elements in the vector |
vector::max_size(); | Returns the maximum number of elements the vector can store |
vector::capacity(); | Returns the storage space allocated to the vector |
vector::resize(); | Changes the size of the vector |
vector::empty(); | Returns true if vector is empty otherwise false |
vector::reserve(no of elements); | Sends a request to reserve at least the space that can store a certain number of elements specified. |
Accessing Elements
Function | Description |
---|---|
vector::front(); | Returns first element of the vector |
vector::back(); | Returns last element of the vector |
vector::data(); | Returns the pointer to the array implemented internally by vector |
vector::at(i); | Accesses the element stored at index ’i’ |
vector_name[i]; | Accesses the element stored at index ’i’ |
Searching
Function | Description |
---|---|
find(start_iterator, end_iterator, value); | Returns vector::end() if value is not present in the vector. If it is present then its iterator is returned; |
distance(start_iterator, end_iterator); | Returns the distance between the two iterators |
advance(iterator, distance); | It advances the given iterator by the distance given. |
Conclusion
It can be concluded that vectors offer us a lot more than arrays and in order to excel in programming, one has to study the STL, especially the vector class.
Reasons for such an abundant usage of vectors are as follows :
- Dynamic nature (the size of a vector can be adjusted accordingly).
- Since vectors offer us dynamic arrays. We are able to have unique-sized arrays even in multidimensional arrays.
- There are multiple ways to copy one vector to another.
- Easy to pass as an argument.
- Easy initialisation with constant values.
- Multiple member functions for user's convenience.
- Easy to clear the vector for saving memory.
A vector is always a better option over arrays and linked lists in storing and managing bulky and dynamic data in our program.