Python Program to Find Factorial of a Number
Learn via video course
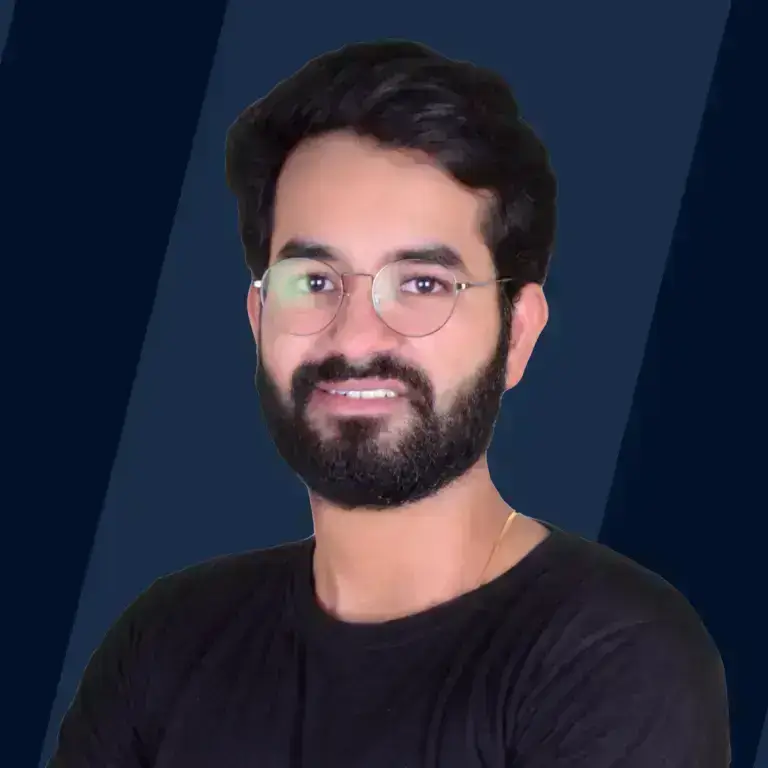
Overview
Factorial of a non-negative integer, is the multiplication of all integers smaller than or equal to itself. It is denoted using the symbol "!". It is commonly used in programming while solving problems and is a must-have tool in our programming toolkit.
Introduction to Python Factorial Program
We all must have heard or read about the word ‘Factorial’ of a number in our school and might have wondered what it meant.
Let us understand it with the help of an activity. In the picture given below, we have three beakers having different colors. Let us arrange them differently and see how many possible ways we can arrange them.
We see that there are, in total, six ways of arranging the given three beakers differently. Now doing such a long process of jotting down all possible arrangements is tedious and time-consuming.
For this, we can observe that the first beaker can be placed in any of the three positions, then out of the two positions remaining, we can put the second beaker in any of the two positions, and for the last beaker, we just have one place remaining.
The diagram below explains how we can find the number of arrangements using this logic.
It is called ‘Factorial’, i.e., the product of all the numbers from 1 to the given number. So if the number is 7, the factorial of 7 will be calculated as a product of all numbers from 1 to 7.
Now let us see what exactly the word ‘Factorial’ means.
What is Factorial?
The factorial is the product of all the numbers less than or equal to the given number till 1. For example, the factorial of 7 will be 7\*6\*5\*4\*3\*2\*1, which is 5040.
The factorial of a number is denoted by an exclamation sign (!) in Mathematics. For example, the factorial of 7 is denoted as 7!, similarly the factorial of any number N is denoted as N!
Algorithm for the Factorial Program in Python
For any number N greater than 0, we can write:
Note:
For N = 0, factorial is 1. For N < 0, i.e. for negative numbers, factorial does not exist.
Examples
Factorial of 7
Factorial of 7 is 5040.
Factorial of 10
Factorial of 10 is 3628800.
Factorial of 5
Factorial of 5 is 120.
Factorial of 0
Factorial of 0 cannot be calculated by the formula given above since that is for positive numbers. So, the factorial of 0 is 1 i.e. .
Python Program to Find Factorial of a Number
There are two ways of implementing the factorial program in Python, i.e., using a loop and recursion. Firstly we will see the code with recursion and then use a loop.
Using Recursion
In the following set of programs, we will use recursion to find the factorial of a number. Now recursion is a process in which a function calls itself for smaller or simpler input values.
For example, when calculating the factorial of 4, we write: . If we see closely, we will find that is the factorial of 3. Hence 4! Can be written as,
Thus to generalise this equation, we can write , for , i.e. for all positive values.
For N = 0, we already know that 0! = 1, this condition will be the base case in our recursion code. We can also see that in recursion, we will call for (N-1) factorial, and assuming that answer is correct, we will calculate the answer for N factorial.
Now, (N-1) factorial will make a call on (N-2) factorial to get the answer, and this will go on until we reach 0 i.e. our base case. Finally, using the answer found at each step, we will calculate our final answer, i.e., factorial of N.
Hence to summarize:
For N < 0, Factorial does not exist
For N = 0, factorial(0) = 1
For N > 0, factorial(N) = N * factorial(N-1)
Input:
Output:
Explanation -
Here we want to find the factorial of 4, and we see the function named ‘fact’ recursively calls itself until we reach 0. And since we already know the factorial of 0. We will use this value to calculate the factorial of 1, 2, and so on up to 4.
We can understand the working better with the diagram given below:
2. Input:
Output:
Explanation- Since the value of the number is negative (i.e. N < 0).
3. Input:
Output:
Explanation:
Since the value of the number is 0, the control goes to the elif statement and 1 is returned straight away without making any recursive calls.
Using For Loop
In the following set of programs, we will see how to calculate the factorial of a number using the help of a for-loop in Python. We will run the loop from 1 to the given number. At each step, the value of the loop control variable (i.e., i) gets multiplied by the variable fact, which stores the value of the factorial of the given number.
For non-negative numbers
1. Input:
Output:
Explanation:
The loop runs from 1 to num i.e. 7, and every time ‘i’ gets multiplied by the variable ‘fact’. So, the corresponding values of ‘i’ and ‘fact’ at each iteration are
i = 1, fact = 1
i = 2, fact = 2
i = 3, fact = 6
i = 4, fact = 24
i = 5, fact = 120
i = 6, fact = 720
i = 7, fact = 5040
Using if-else Statement (For nonnegative integers as well)
In the following programs, we will add an if-else statement to handle the cases where the value of the given number is negative. Apart from that, the logic for calculating the factorial will be the same as used above in the case of for-loop.
1. Input:
Output:
2. Input:
Output:
Explanation:
The control goes to the elif statement, and 1 gets printed which is the value of 0!.
3. Input:
Output:
Using Math Module
In the following program, we will be using the factorial() function, which is present in the Math module in python to calculate the factorial of a given number.
This function accepts only a positive integer value, not a negative one. Also, this method accepts integer values not working with float values. If the value is negative, float, string or any data type other than an integer, it throws a “ValueError”.
1. Input:
Output:
2. Input:
Output:
3. Input:
Output:
Using Ternary Operator
In the following programs, we will use the same recursive code that we have seen above, the difference being that instead of the if-else conditional statement, we will use the ternary operator in Python, which is also a type of conditional statement.
In a ternary statement, the format is like this:
[on_true] if [expression] else [on_false]
First, we write the work to be done when the expression is true, and then we write the work to be done in the else case, i.e., when the first expression is false.
In the given code below, we can see that if the value of ‘num’ is 0 or 1, the first expression becomes true, and value 1 is returned. If the value of num is greater than 1, then a similar recursive call is made, as we have already seen and understood above.
The recursive calls are made till we reach a value whose factorial value is already known, i.e., either 0 or 1, from where the values are calculated. Then the final factorial value of the number is returned.
1. Input:
Output:
2. Input:
Output:
Explanation -
The control goes to the elif statement, and 1 gets printed which is the value of 0!.
3. Input:
Output:
Applications of Factorial in Python
There are tons of applications when it comes to using a factorial. Anytime you want to know anything about combinations and rearrangements, chances are you’ll find the use of factorial.
Besides that, they are extensively used in various other concepts of Mathematics, like Probability, Number Theory, Statistics, Algebra, Calculus, etc. Factorials are also very useful for facilitating expression manipulation.
Conclusion
Now that we have seen everything regarding the factorial of a number let us revise a few points.
- Factorial always exists for non-negative numbers, i.e. for all N >= 0.
- Factorial of a number is always a positive value, i.e. factorial is always > 0.
- Factorial of 0 is taken to be 1.
- Factorial of any number N > 0, can be defined as: