init Function in Python
Learn via video course
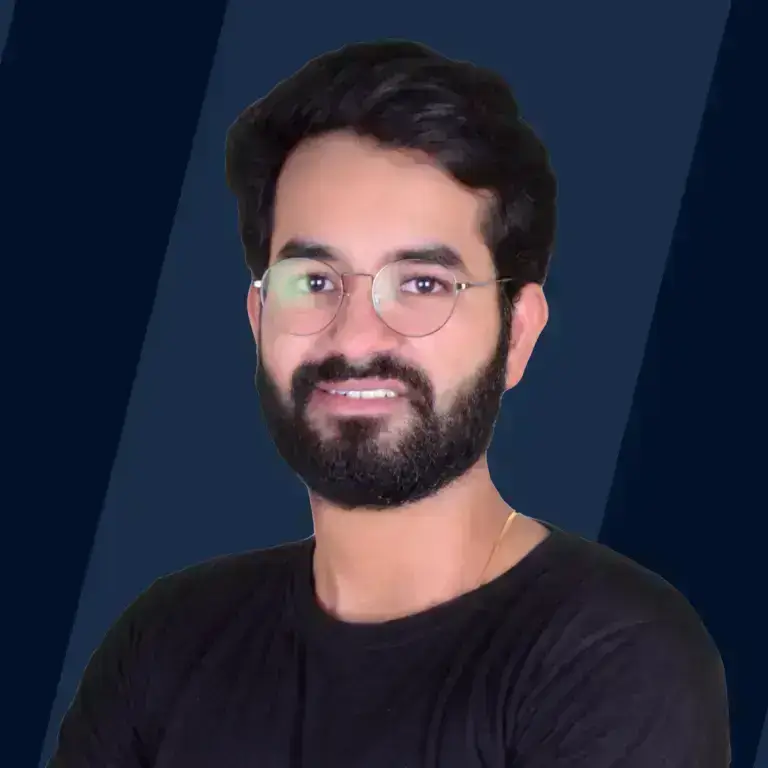
Overview
In an object-oriented approach, the __init__ method is the Python equivalent of the C++ constructor. Every time an object is created from a class, the __init__function is called. The __init__ method only allows the class to initialize the object's attributes. It is only used within classes.
Scope
- In this article, we will learn about the __init__ method in Python
- We will also learn various applications of the __init__ method in Python, along with examples and codes for the same.
Introduction to __init__ Function in Python
Python is an object-oriented language. This means that everything in Python is an object. This feature of Python makes it one of the most preferred languages to learn. Let’s first clarify some basics about Object Oriented Programming, commonly called OOP.
Let’s say you are making a program that stores information about cars. You will create a class of name Car and then create objects of different cars. When creating a class object, we pass different things to it. In the case of cars, it will be company model, year of manufacture, etc. So, inside the class, we need a function that can assign all the arguments passed while creating the object to the newly created object. Or you might want to print something whenever a new car is added to your program.
There is a method called __init__() for this task. This method is called automatically whenever a new object of a class is created. This type of function is also known as the constructor function. A constructor function is a function that is called every time a new class object is created.
Here is an example of a class Car that will clarify the functioning of __init__().
Code:
Output:
Explanation:
You can see in the output the order of print statements that __init__ is called first.
__init__ is a magic method called Dunder Methods (These are special methods whose invocation happens internally, and they start and end with double underscores). Other examples for magic methods are: __add__, __len__, __str__, etc.
Syntax of __init__ function in Python
Syntax:
__init__() function is defined just like a regular python function. Self must be the first argument. After that, you can pass arguments of your requirements. The logic of the function comes in the function definition part.
What is __init__ function in Python
As the above example explains, __init__ is a special python method that runs whenever a new object is created. These types of functions are used to initialize the attributes of that class, E.g., if the class is Person, then the attributes will be name, age, etc.
In other Object-Oriented Languages(like C++, and Java), these types of functions are called constructors. Constructors are used to initialize the data members of the class when an object is created. Python uses the __init__ method as a constructor to initialize the data members when an object is created for the class. Let’s look at some examples which will help in understanding __init__ better.
Code:
Output:
Explanation:
In the above example, a class Trip is created, and an object ‘T’ is created to invoke the methods and attributes. As soon as the object is created,__init__ method is initialized with values India and London which are assigned to to_city and from_city respectively. The self keyword represents the current instance of the object. So when the holiday() method is run, it prints out the values of from_city and to_city, which are London and India, respectively.
Code:
Output:
Explanation:
In the above example, object ‘I’ is created to invoke the methods of class Insects, and the __init__ method initializes with values ‘6’ and ‘2’, which are assigned to legs and wings, respectively. When the looks() method is called, the value of legs and wings is printed out to the console.
Calling __init__ Function in Python
Generally, we do not call the init manually. It is automatically called when a new object is created. init method is only called manually in the case of inheritance. We will discuss using init in inheritance in the next section. If you want, you can call the init() directly, here is how you can do it
Code:
Output:
Explanation:
In the above example, first we set the value of str linked to obj to ‘hello’ and then calling the __init__() directly we set the value of str to ‘Python’. In this way the __init__() method can be called directly.
Inheritance of __init__ Method in python
Inheritance is a very important concept in OOPs (Object-Oriented Programming). Let’s first understand inheritance in general terms and then we will worry about Python.
You might have seen some families where all the members have blue eyeballs. Or somewhere, all family members have curly hair. This happens because the genetic information from parents is passed on to the children through a natural process which we call inheritance.
This same concept is used when writing Object Oriented code. Here the parents are called the base class and the children are called the derived class.
The derived class can access the properties and methods of their parent class. In other words, the child class inherits all the methods and properties of their parent class and can add more methods and properties which are unique to them(child class) only. Inside a child class __init__() of the parent class can be called to create object and assign values. Here is an example:
Code:
Output:
Explanation:
In the above example, when the object of the Child class is created, __init__() of the Child class is called, which first calls the __init__() of the ParentClass. __init__() of the ParentClass prints out ‘Parent Class’ then print inside the Child class is executed.
Conclusion
This article had a lot of information about the init method in python. Here is a quick summary
- __init__() is a special python method that runs when an object of a class is created.
- __init__() function is mostly used for assigning values to newly created objects.
- __init__() is a magic method which means it is called automatically by Python
- __init__() can also be invoked manually.
- __init__() also supports inheritance.