Inline Functions in C++
Learn via video course
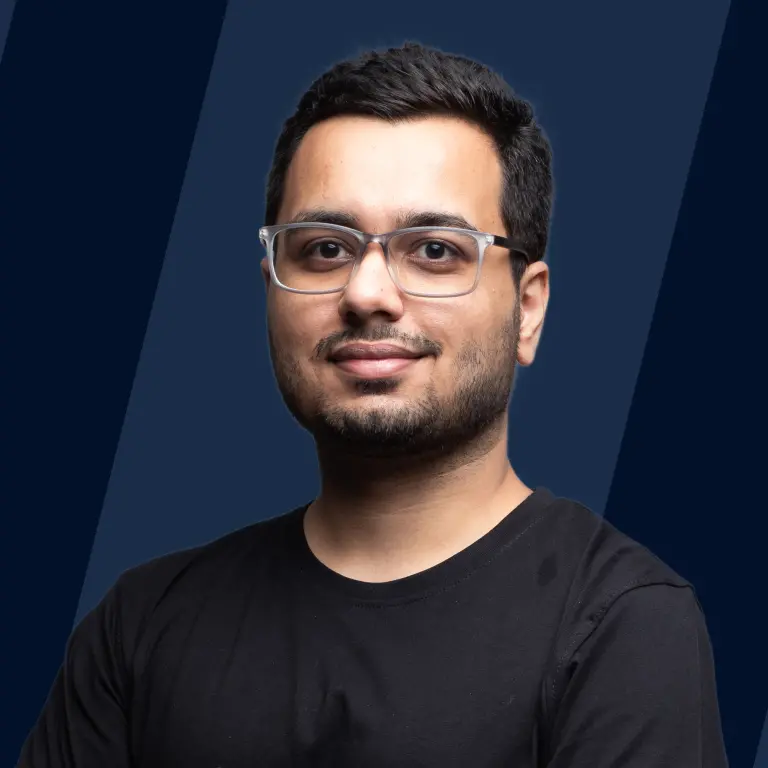
Overview
Inline Function is one of the most important feature of C++. Using the C++ inline function, the function code is compiled when the program is executed. This saves time in calling the function repetitively as it is available with the calling program. Thereby increasing the execution speed and reducing execution time to a great extent.
Scope
- This article covers the concept of inline function in C++
- It covers advantages, disadvantages and use of inline function in C++
Introduction
Imagine yourself as one of the qualifiers of ICPC(International Collegiate Programming Contest) and your team is competing to be in the top position. The questions are trickier and you have to optimize your code to perform at its best.
You would never wish to miss the opportunity to compete with the World Finals. Do you?
The inline function in C++ would just be a beginning to your world of competing with thousands of programmers. Let us look at the whereabouts of this function in detail.
What is an Inline Function in C++?
The C++ inline function is called at the compile time by the compiler thus substituting the inline code at the point where the inline function is called.
This optimization feature reduces the overhead function calls by replacing the function call with the function code.
Sounds complicated?
Understand it as a situation where you would have to call a function multiple times, let’s take cout as a reference.
In the program, you would be calling cout continually which will increase execution time. To overcome this, define an inline function in the header and call as much as you require.
Left Side
Right Side
The syntax for defining a function inline is:
Syntax:
Example:
Explanation: The above code declares inline functions outside the body of the program. Simple addition and subtraction of two numbers display the use of the C++ inline function.
Difference between the normal function and the inline function
In C++, a normal function and an inline function serve a similar purpose in that both are used to encapsulate a block of code into a single unit that can be reused throughout a program. However, there are some key differences between the two:
- Definition: A normal function is defined outside of the class or the structure whereas an inline function is defined inside the class or the structure.
- Execution: When a normal function is called, the program jumps to the function's memory location, executes the code within the function, and then returns control to the calling function. With an inline function, the code for the function is directly inserted into the calling function at compile time. This can result in faster execution time because there is no function call overhead.
- Usage: Normal functions are generally used for more complex operations or computations, while inline functions are typically used for small, frequently used operations. Inline functions are particularly useful when the function is called multiple times within a loop, for example.
- Restrictions: There are certain restrictions on what can be done in an inline function. For example, inline functions cannot have static variables, cannot contain a loop that isn't a compile-time constant, and the entire function definition must be visible in every file where it is used.
Overall, the choice between a normal function and an inline function depends on the specific requirements of the program and the intended use of the function.
Advantages of using Inline Function in C++
To list a few, these are some of the reasons which will make you use this function in your programs.
- The program’s execution speed increases as there are no overhead calls.
- The function saves the overhead of push/pop variables on the stack during function calls.
- The overhead of the return call is saved by a C++ inline function.
- The inline function can be saved in a header file saving time and making program execution faster.
- The locality of reference is increased by the utilization of the instruction cache. As the number of cache lines which are used to store the code decreases, the performance of CPU-bound applications increases, pushing it to run faster.
Disadvantages of using Inline Function in C++
As there is life with room for mistakes, the function comes in with a bunch of cons that need to be taken care of.
- For a long piece of code, it will increase the executable size.
- As the inline function is called at the compile time, a small change in inline code will make your way to recompile all to get it updated.
- The use of excess C++ inline function can lower the instruction cache hit rate, resulting in a reduced speed of instruction from the cache to primary memory.
- The embedded systems may not have inline functions as the code size is more important than their speed.
- The more the number of inline functions, the more the executable size of a file that causes thrashing of memory and degrades the computer’s performance.
Use of Inline Function in C++ Class
The functions which are declared in a Class are predefined inline.
For a better approach, the programmer should declare the function inside the class and define the function outside with an inline keyword.
Example:
Explanation: The prototype of the function is declared inside the class and explained outside with the use of an inline function in C++.
Why do we need an inline function in C++?
A function call transfers the control from the calling programs to the called function.
The tedious process of storing memory address, following function call, loading, copying, executing, and returning the value to the function heightens up the time and occupies memory.
Using the C++ inline function, the function code is compiled when the program is executed. This saves time in calling the function repetitively as it is available with the calling program. Thereby increasing the execution speed and reducing execution time to a great extent.
When to use an Inline Function in C++?
There are various use cases of C++ inline function out of which a few are listed below.
- Always choose inline functions over macros in your program.
- When performance is the major factor of a program, choose inline functions to speed up the process.
- Refrain from using the inline keyword inside the class with the function definition.
The inline function acts as a request to the compiler which could be overlooked under certain circumstances:
- The inline function has a loop or static variables.
- The inline function in C++ is recursive or uses switch statements,
- The inline function does not have a return statement.
What is wrong with the macro?
- C++ macros are almost never necessary and are error-prone, according to Bjarne Stroustrup, the creator of C++. Macros cannot access private members of a class and are managed by the preprocessor, while inline functions are managed by the C++ compiler.
- It is recommended to always use inline functions instead of macros. Inline functions are checked by the C++ compiler for argument types and necessary conversions, while macros are not capable of doing so.
- All functions defined inside a class are implicitly inline and the C++ compiler will perform inline calls of these functions. However, if the function is virtual, it cannot be performed during compilation because the call to a virtual function is resolved at runtime.
- Inline functions are only useful if the time spent during a function call is more compared to the function body execution time. For example, a function that performs input-output (I/O) operations should not be defined as inline.
- Inline functions are a valuable feature of C++, but their appropriate use can provide performance enhancement. It is better to keep inline functions as small as possible and not make every function inline, as arbitrary use of inline functions cannot provide better results.
Example:
Output:
Sum = 15
In the above example, the add() function is defined as inline. When the add() function is called, the C++ compiler replaces the function call with the actual function code, resulting in faster execution time.
Conclusion
For easy readability and faster execution of the programs, the inline function in C++ is used by the programmers.
Clearly, the pros and cons weigh equally, yet many programmers prefer using inline functions in the header when competing globally.
That’s all for now folks!
Thanks for reading.