List in Java
Learn via video course
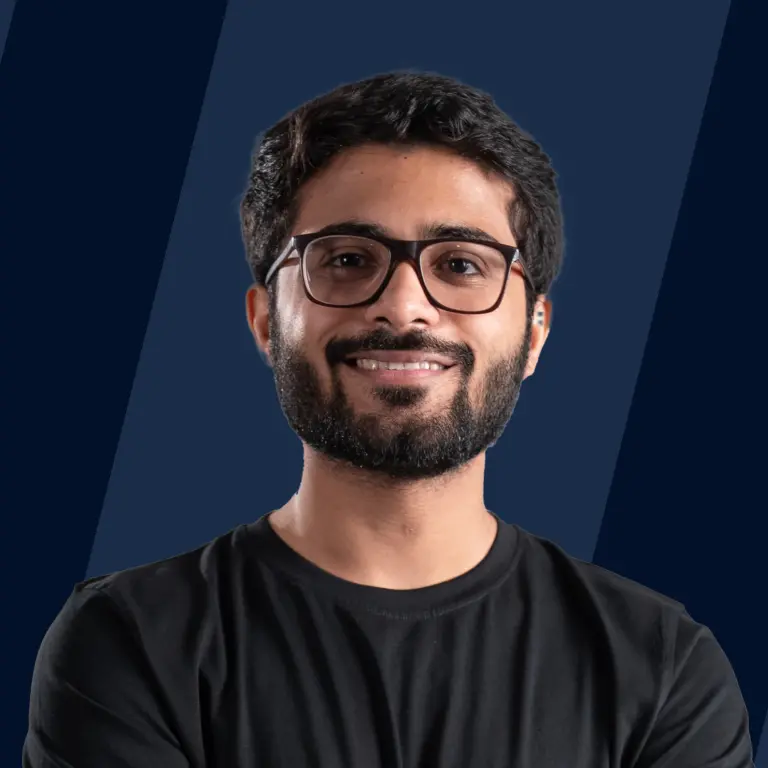
Overview
List in Java provide the dynamic as well as an ordered collection of the user-defined or predefined elements. Lists in Java implement the List interface. Lists in Java can store null or duplicate elements.
The internal working of the list in Java is based on the indexing mechanism.
The classes such as ArrayList, LinkedList, vector, stack,copyonwriteArrayList, and Abstract list implement the features of the list by implementing the List interface.
Introduction to Java List
The main disadvantage of the array data structure is fixed-length, which means an array can store only a limited count of elements and will cause an error if we try to enter an extra element.
Now how can we solve this problem? We want such types of data structures that are not based on the fixed-length property, and we can add as many elements to the data structure.
Yes, in Java there are different types of classes like ArrayList, LinkedList, Vector, Stack, and CopyOnWriteArrayList that allow dynamic insertion of the elements as well as the elements are stored in order. Along with these properties, these classes provide many inbuilt methods to increase the efficiency of the programmer.
Now you might be wondering how these classes store the elements in an ordered way! To get the answer to this question, we have to understand the hierarchy of these classes.
Let's have a look at the hierarchy of these classes.
We can see all classes implement the List interface, and this List interface contains the signature of many methods that help us to design many features in the class, like maintaining the order, and insertion of the element at the particular index, etc. These classes use the abstract methods of the List interface to design the structure of its class.
How to Create a List in Java?
As we know very well we cannot create an object of the interface and a List is the interface that contains all abstract methods of a list. So, how can we create a List in Java?
There are different types of classes in Java that implement the List interface. The classes are ArrayList, vector, Stack, LinkedList, etc. We can take the help of these classes to create a list in Java.
There are two ways to create a list in Java:
- Using the List interface (Programming to the interface).
- Without using the List interface (Concrete Implementation).
Let's discuss each method one by one.
- Using the List interface: This method is also known as Programming to interface because, in this method, we will take the help of the List interface to create a list. Because all the lists in Java implement the abstract methods of the List interface, we can use them to access the implementation of these methods for creating a list in Java.
Let's see an example for better understanding.
In the below snippet, we are creating a list using the interface List that contains the Object class objects as an element by default.
In the above way, we can only create a list for storing the objects of the Object class, but how we can make a list for storing the elements of other data types such as int, float, etc.
We will take the help of the generics, and we know very well that generics help to handle the different types of data types in a single implementation, and they are used for storing the references; that's why the list cannot be used for storing the primitive datatypes elements like int, float, etc.
In their place of them, we will take the help of the respective wrapper class of primitive datatypes and can create a list.
The above snippet is used for creating the list of the Integer reference using multiple classes.
- Without using the List interface (Concrete Implementation).
In this method, we simply use the object of the class that implements the List interface, Classes may have the same or different implementation of the List's abstract methods.
We will take the help of the object of these classes for creating a list in Java.
Let's understand this method using an example.
In the above snippet, we are using the concrete implementation of the list in java.
Now, you might be wondering what is the main difference between this implementation. This is the question for you :).
Example of Java List
Example 1: Using The asList() Method
The asList() method is used to convert the specialized array into the list(ArrayList, vector, stack, LinkedList).
Note: The type of an array that needs to be converted into a list must be a Wrapper class, we have already discussed the reason in the above section.
Syntax:
Parameter: This method takes varargs as a parameter means a variable number of arguments.
Return type: This method returns a list.
Let's understand this method using an example.
Output:
The Resultant list is the type of the ArrayList.
Example 2: Using List.add()
The add() method of the list classes is used for adding the user-defined or wrapper class objects to the existing list. Syntax:
Return type: This method returns true, after every new addition of the new elements.
Let's understand this method using an example
Output:
Example 3: Using Collection Methods
- Collection interface is the parent interface of the List interface.
The add(E element) of the Collection interface is used for adding the element to the list.
Because the Collection interface contains the abstract method for adding the element, and this method is implemented by all the list classes that's why it can be used for creating a list.
Let's understand this method using an example.
Output:
Example 4: Using Java 9 List.of() Method
The List.of() takes varargs as an argument and returns the immutable list.
Syntax:
Parameter: This method takes a varargs as a parameter.
Return: This method returns a list.
Output:
Java List Methods
Methods | Description |
---|---|
void add(int index,E) | This method of List interface is used for inserting an element at the particular index of the list |
boolean add(E e) | This method is used for appending the element at the end of the list and returns true if the element is successfully added. |
void clear() | This method is used to remove all the elements from the list. |
boolean equals(Object o) | This method is used for the comparison of two objects. |
int hashcode | This method is used to get the hash value of an object. |
E get(int index_value) | This method is used to fetch an element that is present at the given index value in the list. |
boolean isEmpty() | This method is used to determine whether the list is empty or not, if empty this method returns true, otherwise false. |
int lastIndexOf(E e) | This method is used to get the last index value of the given object. If the object is not present in the list, this method returns -1. |
boolean contains(Object E) | This method is used to check whether the list contains specialized objects in the list or not, if present this method returns true, otherwise returns false. |
int indexOf(Object E) | This method is used to fetch the first index position of the given object in the list, if the object is not present, This method returns -1. |
List remove(int index) | This method is used for removing the element or object present at the given index position. An exception will occur if we provide an invalid index value. |
boolean remove(Object E) | This method is used to remove an object from the list and returns a boolean value. |
List set(int index, Object) | This method is used to set an object at the given index position in the list. |
int size() | This method is used to determine the size of the List. The return type of this method is int. |
Spliterator<E> spliterator() | This method is used to create a spliterator over the elements in a list. |
boolean addAll(Collection<? extends E> c) | This method is used for appending the particular collection at the end of the existing list. |
List.toArray() | This method is used to return the array containing all elements of the list in an ordered way. |
These are some methods of the List interface, and many classes implement these methods to perform their respective operations.
Operations in a List interface
Let's understand some operations of the list interface methods.
a. Adding Elements to List Using add() method
The List interface contains an abstract method i.e add() method, that is implemented by multiple classes and used for adding the elements in the list.
Let's understand adding elements to the list using add() method.
Output:
In the above snippet, we are adding the elements in the list using the add() method of the ArrayList class.
b. Updating Elements in List class using set() method
The set() method of the List interface is used for updating elements in the list class. We have already discussed the syntax and return type of this method in the above section. Please have a look at the table for a better understanding.
Output:
In the above snippet, we are updating the String at index 1 with the "HelloScaler" String in the list.
c. Removing Elements Using remove() Method
The remove() method of the List interface is used to remove elements from the list. This method takes the index of the element that needs to be removed or an object of the element.
Let's understand this method using an example.
Output:
In the above snippet, we are removing the elements from the given list using the indexing method and using the reference method.
How to convert Array to List in Java?
The Arrays.asList() method is used to convert Array to list in java. This method can only convert the array of the type wrapper class, not a primitive type. We have already discussed the reason behind this in the above section.
Let's understand how to convert array to list in java using this method.
Output:
The Resultant list is the type of the ArrayList.
How to Convert List to Array in Java?
The toArray() method of the list class that is implemented from the List interface is used to convert the list to Array in java.
This method returns the array of an object class, and in order to perform further operations on the array, We have to explicitly pass an array in this method of wrapper class type.
let's understand this method using an example.
Output:
How to do Sort List in Java?
The collections.sort() method of the collection interface is used to sort the list in java such as ArrayList, vector, Stack, LinkedList. Syntax
Parameters: This method takes only one parameter i.e the list that needs to be sorted.
Return type: This method doesn't return anything.
Let's understand this method using an example.
Output:
In the above snippet, we are sorting the elements of the list using the collections.sort() method.
Classes Association with a List Interface
Let's discuss the classes that are implementing the List interface. First, please refer to the below picture for a better understanding of the hierarchy of the List interface.
We can see clearly, the List interface is implemented directly or indirectly by the list classes or abstract class. However, the internal working of these classes is different because due to the separate functions of the implemented methods, some classes are used immutable lists for storing the elements or some use mutable lists.
Let's dicuss about these classes one by one.
AbstractList
This is an abstract class that implements the List interface and is further extended by the many classes like ArrayList and Vector class.
The main use of the AbstractList class is used to provide the functionality of abstract methods of the List interface to reduce the implementation time of these methods again and again in the other classes.
CopyOnWriteArrayList
This class also extends the List interface, and it is the thread-safe variant of the ArrayList class in which all mutative operations (add, set, and so on) are implemented by making a fresh copy of the underlying array.
ArrayList
The ArrayList class extends the AbstractList class and implements the List interface. The ArrayList class uses a dynamic array to store the elements.
We can access the elements in the ArrayList in the time complexity. If we want to perform more searching operations than the add or remove operation on the elements, we will prefer the ArrayList because due to the time Complexity of ArrayList for searching the elements.
Vector
The Vector class is the variation of the ArrayList class, but an object of the Vector class is synchronized which means multiple threads cannot access the object of the vector at the same time, that's why it is slower than the ArrayList class.
Stack
The Stack class extends the properties and behavior of the Vector class but the Stack class is based on the LIFO property which means Last-in-First-Out elements.
The pop() and push() methods of the Stack class are mostly used for performing LIFO operations. The many algorithms of the CPU is based on the Stack operation, i.e., LIFO.
LinkedList
The LinkedList class implements the deque and the List interface. It is used to store the elements in the non-contiguously form that's why adding and removing elements in the LinkedList is much easier as compared to other list classes.
Conclusion
- Lists in Java provide the dynamic as well as an ordered collection of the user-defined or predefined elements.
- The List interface is the common interface for all the list classes.
- ArrayList class is efficient in searching for elements, whereas the LinkedList class is efficient for adding and removing elements from the list.
FAQ's
1. What is Arrays.asList() in Java?
This method it used to convert the Array to the list that is ArrayList.
2. What is the toArray() Method in Java?
This method is used to convert the list to the respective wrapper class array.
3. How do you Sort a List in Java?
We can use the Collection.sort() method to sort the list either in descending order or in ascending order.
4. What is the get() method in Java List?
This method is used to fetch an element that is present at the given index value in the list.