Python Comprehensions
Learn via video course
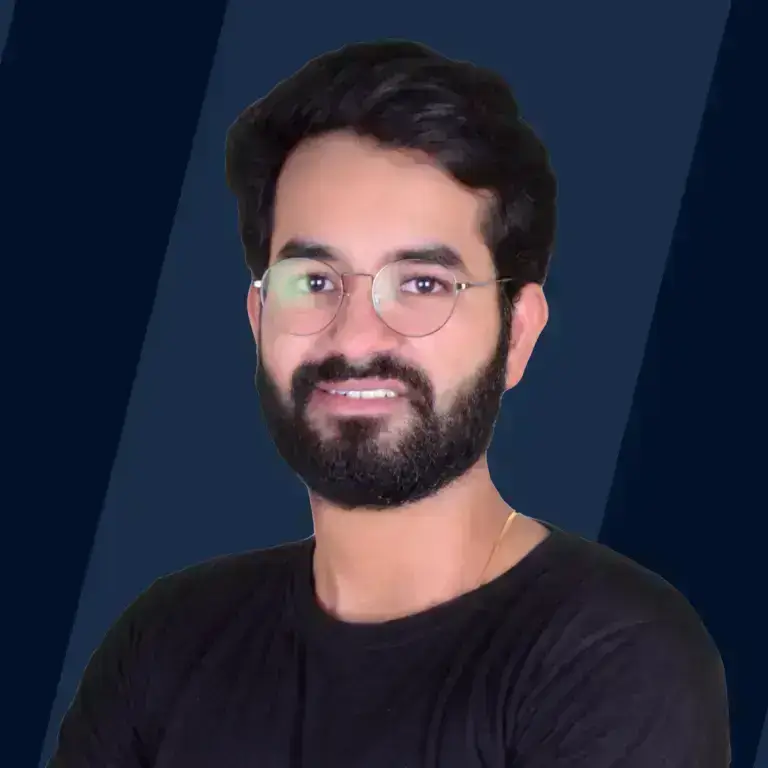
What are Comprehensions in Python?
Imagine you have 10 blue balls in a bowl with numbers embossed on them and a bowl of red paint. You have been endowed with the task of converting these blue balls into red ones. How would you do it?
There are 2 ways of approaching this problem:
- Dip the blue balls individually in order of numbering into the red paint
- Add all the blue balls into the red paint at once
Which of the above methods would be easier? The second one for sure. Now imagine, the balls were an ordered list in Python identified by their color Blue and number embossed on them in the format:
[Initial_of_Colour][Number_Embossed]
For example, the ball numbered 5 will be identified as B5.
Now, suppose that you are asked to change the color of all the balls to red, i.e. the initial used for all the balls will now be R. In Python, we use comprehensions to serve the purpose of updating the entire list all at once.
Comprehensions in Python are used to generate new sequences based on existing sequences. In Python, we have comprehensions for lists, dictionaries, sets, and generators. The type of the existing (original) sequence and the new sequence need not be the same. For instance, you can create a new dictionary from an existing list using comprehensions.
To understand comprehension better, let’s see the above example being depicted with and without the use of comprehension.
Example: Suppose you have been given a list blue_list of balls with each item named by the color of the ball. To create another list with the same number of balls as in the blue_list but with colour red, we do the following:
- Create an empty list called red_list.
- Using a for loop, append the word ‘red’ to the red_list for as many times as there are items in the blue_list.
Output:
The first line of output depicts the blue_list and the second line displays the red_list (which is the item ‘red’ as many times as the length of the blue_list.
Now, what if I want to create the red_list from the blue_list without appending each element. Comprehensions are used to satisfy this purpose. Here, we replace the for loop to append each element to the empty red_list created with a simple statement:
Red_list represents the name of the new list, “red” represents the name of the items in the list, list_item represents the item in the existing list and blue_list is the name of the existing list. The whole code is as shown below:
Output:
Which code do you think is clear and concise? Comprehension, it is. To use comprehensions, you simply replace all the code for the list to be created with a line that creates the list using an expression to compute each item in the new list.
Moving forward, let’s understand the different types of comprehension in Python.
Types of Comprehensions in Python
List comprehensions in Python
In Python, list comprehensions are used to create a new list from an existing one by manipulating its elements.
Syntax:
Here, new_list is the new list being created, expression refers to the expression that would be used to manipulate the elements in the list. It could be a formula or a simple value, item refers to the original element in the list, iterable is the original sequence being used to create new_list, and conditional is an optional parameter used to reduce the length of the new_list, making it shorter than the original sequence. Say you have a list of natural numbers, and you want to create a new list with only even numbers in it. The optional
Considering the given example of converting a list of red balls to red. All we do is create a red_list from the existing blue_list by naming each element as red in the new list.
Output: Considering you have a list of consecutive even numbers and want to create a list of consecutive odd numbers, you can do it by adding 1 to every element of the list. This is achieved by considering the item in even_list as var and then adding 1 to it as shown in the following statement:
The complete code for this is as follows:
Output:
Dictionary comprehensions in Python?
A dictionary in Python holds a key-value pair. Dictionary comprehensions are used to create a new dictionary from an existing sequence of dictionaries or lists by manipulating its elements.
Syntax:
Here, new_dict is the new dictionary being created, the key-value pair refers to the expression that would be used to manipulate the dictionary elements. It could be in the form of a formula or a simple value, item refers to the original element in the iterable, iterable is the original sequence being used to create new_dict, and conditional is an optional parameter used to reduce the length of new_dict, making it shorter than the original sequence. Now let’s understand this better with an example.
Consider a list of dictionary objects containing words and their meanings where each dictionary is in the format:
We aim to create a sequence of dictionaries where each dictionary object is in the format:
To do so, we create a new dictionary using dictionary comprehension as follows:
In the above code, d[‘word’] represents the value held by the key, word and the d[‘meaning’] represents the value held by the key meaning.
Output:
Now, what if you have 2 lists instead of 1? Can you still create a dictionary comprehension out of them? Yes, you can. Consider the following example of 1 list, one containing the months of a year and another consisting of natural numbers up to 12. We aim to create a dictionary where the key is the number of the month, and the value is the month's name. A dictionary using dictionary comprehension for the given purpose would be created as follows:
Output:
Set comprehensions in Python
In Python, set comprehensions are used to create a new set from an existing sequence (set) by manipulating its elements.
Syntax:
Here, new_set is the new set being created, expression refers to the expression that would be used to manipulate the dictionary elements. It could be in the form of a formula or a simple value, item refers to the original element in the iterable, iterable is the original sequence (list or set) being used to create new_set, and conditional is an optional parameter used to reduce the length of new_set, making it shorter than the original sequence. Now let’s understand this better with an example.
Considering the given example of converting a set of red balls to red. All we do is create a red_set from the existing blue_set by naming each element as red in the new set as follows:
The entire code for this example is given below:
Output:
Consider you have a list of consecutive even numbers and want to create a set of consecutive odd numbers. You can do it by adding 1 to every element of the set. This is done by considering each item in the existing set even_set as var and then adding 1 to var as shown below:
The full code for the above example is as follows:
Output:
Generator comprehensions in Python
This is one of the most rarely used and least known comprehensions in Python. In Python, generator comprehensions are used to create a new generator from an existing list by manipulating its elements. A generator in Python is a function that returns an iterator that holds a sequence of values instead of a single value. Generators are usually memory efficient as they allocate memory on the generation of items rather than at the beginning.
Syntax:
Here, new_gen is the new generator being created, expression refers to the expression that would be used to manipulate the dictionary elements. It could be in the form of a formula or a simple value, item refers to the original element in the iterable, iterable is the original list being used to create new_gen, and conditional is an optional parameter used to reduce the length of new_gen, making it shorter than the original sequence. Now let’s understand this better with an example.
Consider you have been given a list of the first 6 even numbers and you want to generate a list consisting of the first 6 odd numbers from it. You would simply add a 1 to the numbers given in the existing list. The same can be coded as follows:
Output:
Nested comprehensions in Python
In Python, comprehensions can be nested in one another but at the cost of the simplicity of code. Nested comprehensions increase the complexity of the code, which is against the basic definition of comprehensions. Thus, their use should be avoided as much as possible and made only in case of dire need.
Let’s understand how the same purpose can be satisfied without comprehensions vs with the use of nested comprehensions.
Suppose we need to create a 3×3 matrix represented by:
A) WITHOUT comprehension in Python: We use the append function by traversing through rows and columns to fill them with the required values.
Code:
Output:
B) WITH Nested Comprehension in Python: This method avoids the use of long code or the append function. In comprehension, the inner loop of the code is represented enclosed in square brackets [ ] before the outer loop.
Code:
Output:
Although the above code seems simple to comprehend, in certain cases nested comprehensions can increase the complexity of writing and confuse the programmer.
Conclusion
- Comprehensions in Python are used to create new sequences from existing ones, and the type of the new and original sequence might not be the same.
- Python Comprehensions are classified into 4 types based on the sequence generated. These are list comprehensions, dictionary comprehensions, set comprehensions, and generator comprehensions.
- Nested comprehensions in Python may increase the complexity of writing code and thus should be used only when needed.
- Python Comprehensions can be used to make the code more readable and also make the code run faster in most cases since Python first allocates memory for the list, and then adds elements.
- Comprehensions in Python are more often used when you want to sophisticatedly filter lists, use conditional logic to create them, or map elements.