Differences between List, Tuple, Set and Dictionary in Python
Learn via video course
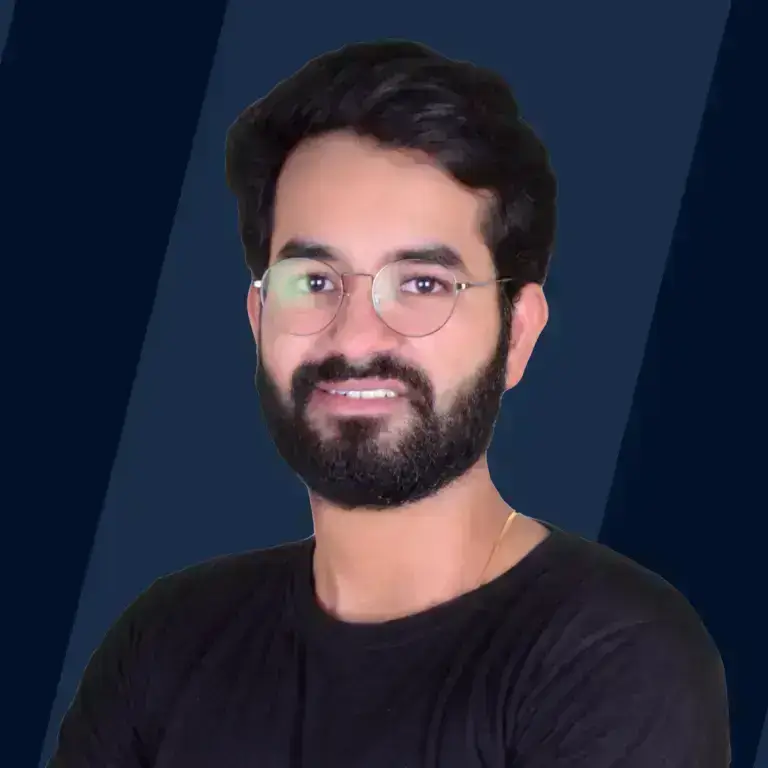
Overview
Data structures in python provide us with a way of storing and arranging data that is easily accessible and modifiable. These data structures include collections. Some of the most commonly used built-in collections are lists, sets, tuples and dictionary.
Scope of article
- In this article, we will discuss about the most commonly used built-in collections in Python, i.e. lists, sets, tuples and dictionary.
- We will discuss the differences between each of these different types of collections.
Introduction
Having a hard time understanding what Lists, tuples, sets and dictionaries are in python? Everyone who works on python at least once has been confused about the differences between them or when to use them. Well, say no more, as we'll break down these Data structure collections' differences and understand them so that we may never forget them!
Let’s take a closer look at what each of these collections are :
List
Lists are one of the most commonly used data structures provided by python; they are a collection of iterable, mutable and ordered data. They can contain duplicate data.
Tuple
Tuples are similar to lists. This collection also has iterable, ordered, and (can contain) repetitive data, just like lists. But unlike lists, tuples are immutable.
Set
Set is another data structure that holds a collection of unordered, iterable and mutable data. But it only contains unique elements.
Dictionary
Unlike all other collection types, dictionaries strictly contain key-value pairs.
- In Python versions < 3.7: is an unordered collection of data.
- In Python v3.1: a new type of dictionary called ‘OrderedDict’ was introduced, which was similar to dictionary in python; the difference was that orderedDict was ordered (as the name suggests)
- In the latest version of Python, i.e. 3.7: Finally, in python 3.7, dictionary is now an ordered collection of key-value pairs. The order is now guaranteed in the insertion order, i.e. the order in which they were inserted.
If you’re questioning if both orderedDict and dict are ordered in python 3.7. Then why are there two implementations? Is there any difference?
We can leave that for when we talk about dictionaries in detail. But until then, we should know what dictionaries are, and their purpose, as that is something that will never change.
Collection VS Features | Mutable | Ordered | Indexing | Duplicate Data |
---|---|---|---|---|
List | ✔ | ✔ | ✔ | ✔ |
Tuple | 𐄂 | ✔ | ✔ | ✔ |
Set | ✔ | 𐄂 | 𐄂 | 𐄂 |
Dictionary | ✔ | ✔ | ✔ | 𐄂 |
NOTE: An ordered collection is when the data structure retains the order in which the data was added, whereas such order is not retained by the data structure in an unordered collection.
- Sets, on one hand, are an unordered collection, whereas a dictionary (in python v3.6 and before) on the other may seem ordered, but it is not. It does retain the keys added to it, but at the same time, accessibility at a specific integer index is not possible; instead, accessibility via the key is possible.
- Each key is unique in a dictionary and acts as an index as you can access values using it. But the order in which keys are stored in a dictionary is not maintained, hence unordered. Whereas python 3.7’s Dictionary and ‘OrderedDict’ introduced in python 3.1 are ordered collections of key-value data as they maintain the insertion order.
Let’s dig deeper into the differences between these collections :
Difference Between List VS Tuple VS Set VS Dictionary in Python
List | Tuple | Set | Dictionary |
---|---|---|---|
Syntax | |||
Syntax includes square brackets [ , ] with ‘,’ separated data. | Syntax includes curved brackets ( , ) with ‘,’ separated data. | Syntax includes curly brackets { , } with ‘,’ separated data. | Syntax includes curly brackets { , } with ‘,’ separated key-value data. |
Order | |||
It is an ordered collection of data. | It is also an ordered collection of data | It is an unordered collection of data. | Ordered collection in Python version 3.7, unordered in Python Version=3.6 |
Duplicate Data | |||
Duplicate data entry is allowed in a List. | Duplicate data entry is allowed in a Tuple. | All elements are unique in a Set. | | Keys are unique, but two different keys CAN have the same value. |
Indexing | |||
Has integer based indexing that has value starting from ‘0’ | Also has integer based indexing that has value starting from ‘0’ | Does NOT have an index based mechanism. | Has a Key based indexing i.e. keys identify the value. |
Adding new element | |||
New Items in a list can be added using the append() method. | Tuples being immutable, contain data with which it was declared, hence no new data can be added to it | The add() method adds an element to a set. | Update() method updates specific key-value pair in a dictionary |
NA | |||
Deleting element | |||
Pop() method allows to delete an element from the list | Being immutable, no data can be popped/deleted from a tuple | Elements can be randomly deleted from a set with pop() method | pop(key) can remove specified key along with its value from a dictionary |
NA | |||
Sorting elements | |||
sort() method sorts the elements of a list | Being immutable, data cannot be sorted in a tuple | Being unordered, a set cannot be sorted | Keys are sorted in a dictionary by using the sorted() method. |
NA | NA | ||
Searching elements | |||
index() allows you to search and return index of first occurrence of a specific element | index() allows you to search and return index of first occurrence of a specific element | An element cannot be searched in an unordered collection | The get(key) method returns value against the specified key. |
NA | |||
Reversing elements | |||
reverse() method allows you to reverse the list i.e. elements now appear in reverse order in the list | Being immutable, reverse() method is not applicable to tuples | Being unordered, reverse() is not advised for sets. | As there is no integer based indexing, hence collection of keys cannot be reversed in a dictionary. |
NA | NA | NA | |
Counting elements | |||
ount() method counts and returns the count of occurrence of a specific element in a list | Count() method counts and returns the count of occurrence of a specific element in a tuple | Count() is not defined for sets | Count() is also not defined for dictionary |
NA | NA | ||
Conclusion
- Lists and Tuples vary in mutability as lists are mutable, whereas tuples are not.
- Set is the only unordered collection in python 3.7.
- Dictionaries store data in the form of key-value pairs in python and remain a controversy when it comes to whether they’re ordered or unordered. As this varies with multiple versions of python. Python v<=3.6 has dictionaries as unordered, but at the same time, orderedDict was introduced in python 3.1, and then finally python 3.7 has both orderedDict and Dictionary and now both are ordered.
Hope this information gives more clarity on what are the differences between list, tuple, set and dictionary in python. And what use cases do they serve and hope it gives you clarity on when to use which collection.