For Else and While Else in Python
Learn via video course
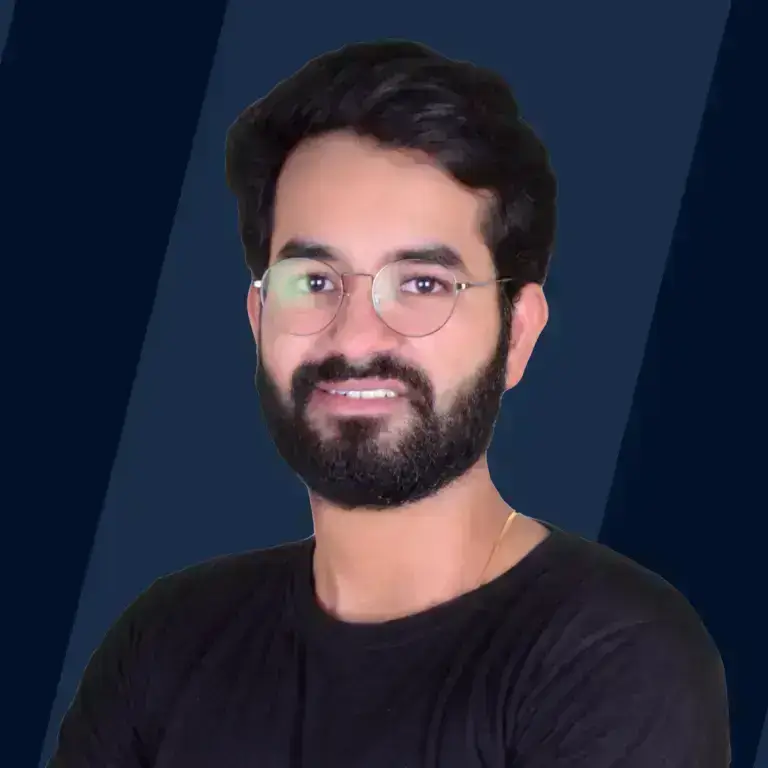
Introduction to For Else and While Else
For loops and while loops are all around us. Are you wondering how? Let me help you with that!
- If you have ever gone to an ATM to withdraw money, you have seen a for loop at play.
- A favourite song that you keep playing on replay? That’s nothing but a loop!
- Even the simple task of unlocking your phone and the number of attempts being limited to 5 is also a for loop.
- The timer on our phone is also a loop! When we start the timer, it will get decremented like a for loop.
- The sequence of day and night is an infinite loop.
Now let’s look into a more technical example. Have you ever wondered how you can check whether an element exists in a list or not?
Let’s see how you can do it using the traditional method which is generally used in every programming language-
Output:
In the above program, the is_grapes variable is a flag variable. After the loop is executed and if that flag variable is false, then it means there are no “grapes” in the fruits list. If the fruit is found, the flag variable becomes true and we stop the loop and break out of it.
Thus, the relevant output is then printed to the console if the conditions are satisfied. So through this code, you can see that using the for loop, we have traversed the entire list of fruits. Using a for loop made our task easier since it's more convenient than writing the same statement again and again.
The traditional method which we have just discussed is useful enough but if you want to make it more elegant then you can use this feature of Python- for-else/ while-else. We will see its use practically after covering the basic concepts of this topic.
Python has this unique feature and concept of for-else and while-else. There are many languages, like C, C++, and Java which do not support this feature.
It may look abnormal if you are encountering this for the first time as you might have been habituated with seeing ‘else’ with ‘if’ statement. But as we progress further in this article you will see its ease of usage and the range of its implementations.
What is For Else and While Else in Python?
For-else and while-else are useful features provided by Python. In simple words, you can use the else block just after the for and while loop. Else block will be executed only if the loop isn't terminated by a break statement.
To put it simply, we can say that if a loop is executed successfully without termination then the else block will be executed.
Let’s try to understand this with the below gif -
It is necessary for a loop to complete all its iterations so that the else block can be executed but the else block will be executed only once.
We have understood the concept of the else block in loops (for & while). Now let’s see the syntax-
For-else Syntax in Python
In the above example, for loop will execute n times and then else block will execute.
While-else Syntax in Python
In the above example, while loop will execute until the condition satisfies and then else block will be executed but if the while loop is terminated by the break statement then else block will not execute, we have seen the same as for for-loop.
Scenarios to use For Else and While Else in Python
It is not mandatory or necessary that we must use the for-else and while-else features in our Python programs
We can also write the same program without the feature of for-else and while-else like we do in other programming languages such as C, C++ and Java. We can do an alternate implementation by using a flag variable..
Now, we are going to see three such scenarios where for else and while else are used. These scenarios are as follows:
- To be used in a searching program
- To check the limits
- To handle exceptions
To be Used in a Searching Program
In this scenario, we are going to search for an item without a flag variable which is used in the traditional approach.
So let’s write a program to find a fruit ‘mango’ in the list of fruits using for-else and while-else.
For-Else Program:
Output:
In the above program, we have iterated through the fruit list. We have used an if condition to check if the current iterator is mango or not.
If the value of i (iterator) variable = mango, then the loop will be terminated and the else block will not execute.
But if we do not find the mango in the list, then the if condition will not be executed and our program will not reach the break statement to terminate the loop. Only then the else block will execute.
While-Else Program
Output :
In this code snippet, we have performed searching by utilizing the while else concept . So we have run the while loop on the condition that the control variable is less than the size. We are then checking whether "mango" is present in the list. If it’s present, we are printing “mango found!” else if it’s not present then the relevant message is printed.
To Check The Limits
In this scenario, we are going to check the limit with the help of for-else and while-else.
We will check if all the numbers in the list are present in between the specified limit or not..
For-Else program
Output:
In the above program, you can see a program to check/validate the limits of all the numbers in the list has been implemented without the flag variable using for-else statements.
Using the same approach, one can also implement the same program with the help of while-else just replace for loop with while loop.
To Handle Exceptions
Exception handling is unignorable for programming, and if we use the for-else or while-else features properly, it can be helpful as well.
In the try statement, the else clause will run when there are no exceptions during execution of the try statement, which is a very similar concept to for-else.
Such as the following example:
Output:
In the above program, we can do the corresponding operations in the else block if there is no ZeroDivisionError in the for loop. If we take a closer look at the code snippet, the exception handling try clause within the for loop is dividing 30 by the numbers present in the list. So if the denominator is zero, then the except clause will execute and the relevant error message will be displayed. In the else part, if there is no error, then the relevant message will be printed to the console.
Conclusion
The for-else and while-else features may look abnormal or weird at first but they are very effective concepts. We just need to keep them in mind while implementing the for-else or while-else.
Here are the things you need to remember-
- Else block in for-else or while-else will only execute once when the loop completes all its iteration without termination by break statement
- We saw how we can use for else and while else in a searching program
- We also took a look at how it can be used to break out of loops
- Also, we saw its implementation in exception handling
Happy coding!