What are the Python Random Functions?
Learn via video course
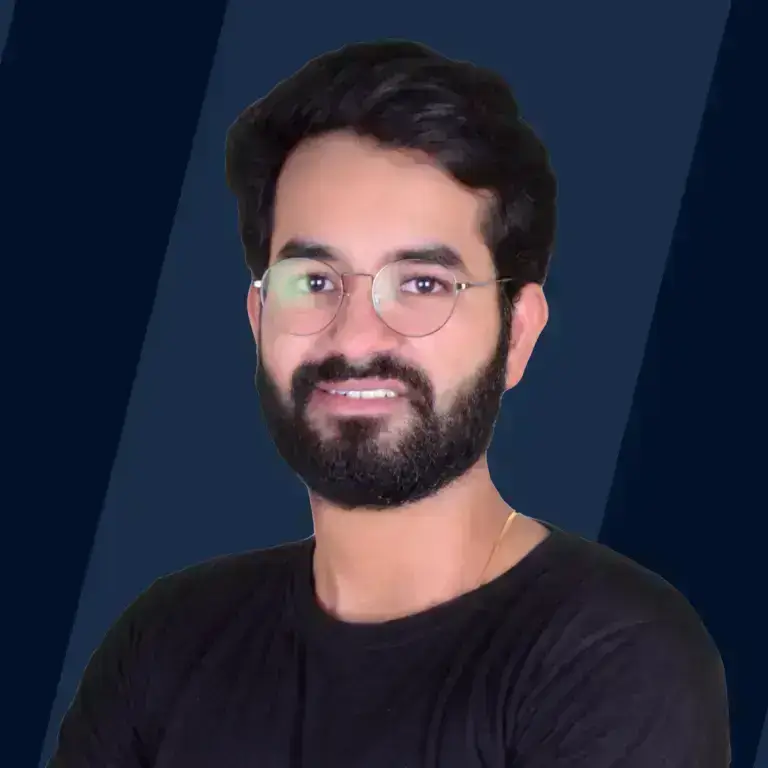
Overview
Python has an in-built random library used to generate random numbers with the help of various in-built functions. For example, we can use random numbers to generate OTPs, lottery tickets, etc.
Introduction to Random Function in Python
Have you ever wondered how a one-time password(OTP) is generated instantly?
For example, a one-time password(OTP) is received whenever a payment is made. It is a random number of some predefined length and can be generated using a random library of python. Read along to know more.
Python has an in-built library for the generation of random numbers. The random library has different functions for generating random numbers in python. The functions of the random library which are used to generate random numbers are:
- randint() function.
- randrange() function
randint() function
The randint() function is an in-built function of a random library in python. randint() function generates the random integers between the two given numbers.
Syntax
The randint function is the inbuilt function of the random library. So we have to import the random library at the start of our code to generate random numbers.
The randint function generates random numbers between 2 numbers (startValue and endValue), which are passed as the parameters to the function. Read along to know more about the parameters.
Parameters
The randint() function accepts 2 parameter for generation of random numbers. Those parameters are:
- startValue: initial value from which random numbers are generated.
- endValue: last value till which random numbers are generated.
Both parameters are mandatory.
The randint() function generate random number from startValue to endValue (both enclusive).
Both of the parameters passed to the randint() function should be integers.
Return Value
The randint() function returns a random number between the start and end values, and both ranges are inclusive.
Two types of errors are generated by passing wrong parameters in the randint() function. These are:
- ValueError: This error occurs if we pass a floating value instead of an integer value in the parameters of the randint() function.
- TypeError: This error occurs if we pass any value other than the numeric value in the parameters of the randint() function.
randrange() function
randrange() function is an in-built function of the random library in python. The randrange() function generates the random number between the range of 2 numbers. All of the parameters are explained in detail below. Read along to know more.
Syntax
The randrange function is the inbuilt function of the random library. So we have to import the random library at the start of our code to generate random numbers.
The randrange function generates random numbers between 2 numbers (startValue and endValue), which are passed as the parameters to the function. Read along to know more about the parameters.
Parameters
The randrange() function accepts three parameters for generating random numbers.
Those parameters are:
- startValue(optional parameter): initial value from which random numbers are generated.
- endValue: last value till which random numbers are generated.
- steps(optional parameter): value to be increased before the generation of the next random number. The default value of steps is 1.
All parameters passed to the randrange() function should be integers.
Return Value
The randrange() function returns a random number between the start and the end value where the start is inclusive while the end is exclusive.
Two types of errors are generated by passing wrong parameters in the randint() function. These are:
- ValueError: This error occurs if we pass a floating value instead of an integer value in the parameters of the randint() function.
- TypeError: This error occurs if we pass any value other than the numeric value in the parameters of the randint() function.
Generating a Random Single Number
We will now create a single random number using the randint function.
Code
Output
Explanation
- We import the random library of python for generating the random number.
- After that, we assign values to the startValue and endValue, which are then passed to the randint() function.
- Then, we call the randint function of the random library to generate a random number.
- Finally, we stored that random number to a variable and printed it to get our random number as an output.
Generating Number in Range
We will now create the random number between a range of two numbers using the randrange function.
Code
Output
Explanation
- We import the random library of python for generating the random number.
- After that, we assign values to the startValue, endValue, and steps which are then passed to the randrange() function.
- Then, we call the randrange function of the random library and pass an upper limit and lower limit as the parameters to it.
- Finally, we stored that random number to a variable and printed it to get our random number as an output.
Generating List of Numbers using For Loops
Here we will generate a list of random numbers generated by the randint() function of the random library of python.
Code
Output
Explanation
- We import the random library of python for generating the random number.
- After that, we assign values to the startValue and endValue, which are then passed to the randint() function.
- Then, we assign a value to the number of random numbers we need to generate in our list.
- Then, we use a for loop for generating n random numbers. Inside of which, we append each random number to a list.
- Then, we call the randint function of the random library for generating a random number.
- Finally, we print the list of random numbers to get the output.
Example of Random Function in Python
Suppose you have to generate a 4-digit one-time password(OTP) for verifying a transaction. So, it would be best to use a function that generates a random number quickly and differently each time. The randint() function helps you generate the 4-digit OTP easily. Let's see how this works.
Code
Output
Explanation
- We import the random library of python for generating the random number.
- After that, we assign values to the startValue and endValue, which are then passed to the randint() function.
- Then, we call the randint function of the random library to generate a random number for the generation of OTP.
- Finally, we stored that random number to a variable named OTP and printed it to get our OTP as an output.
Conclusion
- First of all, we get familiar with what is a random number and how they get generated in python.
- Then, we use two different functions(i.e., randint() and randrange()) of the inbuilt python module to generate random numbers.
- After that, we generate a single random number using the randint() function.
- Then, we create a random number within a given range of numbers.
- Afterwards, we create a list of different random numbers.
- Last but not least, we created an OTP of 4-digit using randint() in python.