sleep() in Python
Learn via video course
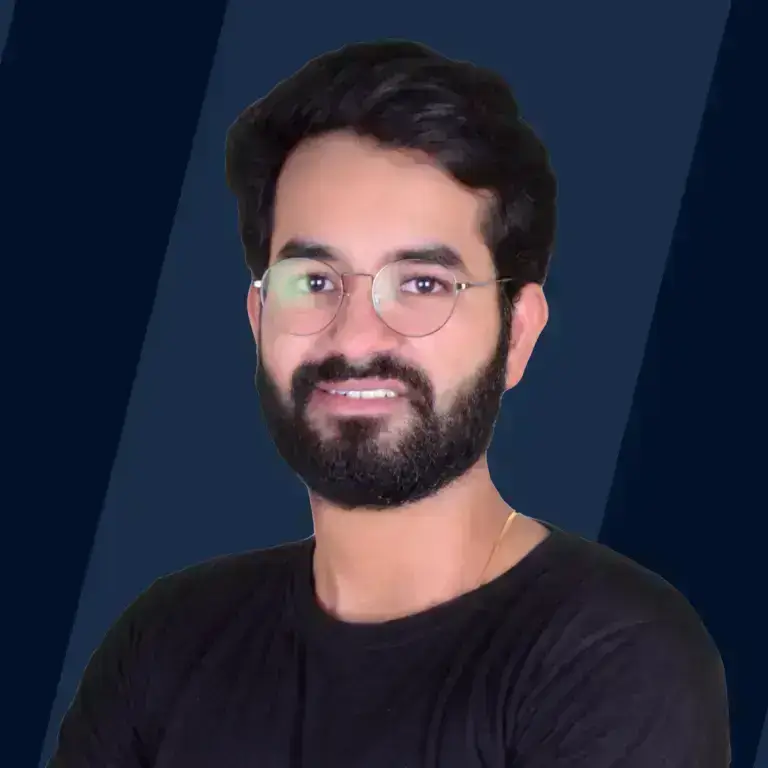
Overview
Python has built-in support for putting our program to sleep. As part of Python's time module, we have a built-in function called . Using the time.sleep() function, we can delay the execution of any program in Python, by any number of seconds. Basically, we can hold the execution of the current thread for some time and perform our desired operations using the sleep() function.
Syntax of sleep() in python
The time module in Python is in-built and we do not need any prior installation before using it. To use the time.sleep() function in Python, we have to first import the time module. Below given is the syntax to import the time module :
Syntax:
Once we have imported the time module, we can use the sleep() function by using time.sleep() in our code. But, you must have noticed one thing, that writing time.sleep() again and again might look redundant, so we can directly import sleep from the time module, and then we can just write sleep() to write our code.
Syntax:
So, now we can only use sleep() in our code.
Let us also look clearly into the parameters that the sleep() function takes.
Parameters of sleep() in python
The time.sleep() function in Python has only one parameter, that is the number of seconds. It represents the delay in seconds, that we can specify for our code. The number of seconds can be either an integer or even a float.
Return Values of sleep() in python
The sleep() function in Python does not return anything in general.
Basic example of sleep() in python
Let us see our first, very basic example of the time.sleep() function in Python.
In the below code, I have added a delay of 3 seconds before the execution of the two print statements.
Code:
Output:
Explanation: In the above code, at the beginning we first print our message, then again after a delay of seconds, our next print statement is executed. So, this is how we can create a delay in our codes using time.sleep().
Alternatively, we could also have directly imported sleep() from the time module, so we did not had to actually use time.sleep() every time, instead, we could simply write sleep(3) to create a delay of 3 seconds. Refer to the code below for a better understanding:
Code:
Output:
what is sleep() in python?
Python's time module provides us with the time.sleep() function. As we already learned above, the time.sleep() function is used to create certain delays in the execution of a program, let us now formally define the sleep() function in Python.
Basically, the sleep() function, in the Python's time module is used to suspend or halt the execution of a program for any given number of seconds.
Hence, the program is paused till that mentioned time, and once the time has passed, our code gets executed automatically. It takes one parameter, that is the delay in number of second. That parameter decides the amount of delay to be made.
Python's time.sleep() function can be used in many projects, also adding a lot of creativity. Also, please note that the time.sleep() method only adds delay to the current thread and does not stop the execution of the program. So, let us understand how can we create delays using the time.sleep() method in Python.
Note: The time.sleep() function only adds delay into our code, and does not stops the execution of any part of the code.
Let us now look into some amazing use cases of time.sleep() function in Python through code examples.
Fact : You can pass the number of seconds as either an integer or even a float value. Providing a float value ensures the very precise amount of delay the code needs to be held for.
:::
More Examples on sleep() in Python
After having briefly understood about what is sleep() in Python, let us now see some useful code examples which can be done through the time.sleep() in Python.
1. Creating Time Delay in List
Creating the time delay in lists is very straightforward because we can put all our elements into the list and then iterate over the list to output their values. Each of the values in the list will be printed after the amount of delay we have provided with our sleep() function.
Let us look into the code for a better understanding :
Code:
Output:
Explanation: In the above code, we have imported the sleep() function from Python's time module. Here, all the messages will be prompted after a delay of 0.7 seconds. So, we can see that creating a delay in a list is a very straightforward task.
Creating Time Delay in Tuple
Creating the time delay in a tuple is very similar to that of creating the delay in a Python list. The only difference will be that, we would be using a tuple for this purpose. Apart from that, we would iterate over the tuple and specify the amount of delay that is expected into our time.sleep() function.
Code:
Output:
Explanation: As you saw in the above code, we did everything similar to what we did in the list. Every name will be printed with the specified delay inside the loop. Also, we have added one more delay before our loop. So, everything goes in order, as per the delay specified.
Creating Multiple Time Delays
Let us now learn how to create multiple time delays using the Python's time.sleep() function. We already saw an example above, where we created multiple time delays. But here we will be adding more delays and creating a suspence game.
Code:
Output:
Explanation: In the above code, we added multiple delays into our code. We created a suspense game where you had to guess something. But the main point is, that we can use time.sleep() any number of times in our code, and inside loops, with print statements, while calling functions, making API calls, etc. There is no limit to which we can create delays in our code using the time.sleep() function in Python.
Creating a Time Delay of 3 minutes in List
Till now, we have learned how to create a delay of a certain number of seconds in our code. But, let us now see, how can we create a delay upto minutes.
Well, you must be aware that there are 60 seconds in a minute! Yeah, that piece of information is sufficient to get started with creating any amount of delays -- be it in seconds, minutes, or hours into our code.
Let us look into the code below to create a delay upto 3 minutes in our code. It will exactly use the method we have stated above, that is, multiplying the expected delay by the number of minutes delay we want in our code.
Code:
Output:
Explanation: In our above code, we created a delay of 3 minutes. That was really simple, isn't it? So, we can just conclude that, to create any delay in minutes, we can just multiply the number of minutes we want to delay, by 60 (the number of seconds). And similarly, for delay in hours, we can just do number of hours * 60 * 60.
Creating a digital clock using sleep in Python
Now that we have studied enough basics about the sleep in Python, it's time for us work on some real applications of time.sleep() in Python.
In this example, we will be creating a digital clock with the help of time.sleep() in Python. Let us look into our code, and then we will understand the operations we performed to create that digital clock.
Code:
Output:
Explanation: In the above code, we created our beautiful digital clock using time.sleep() in Python. So, let us discuss some important imports we have made in this code :
- time : As we already know, to use the sleep() function in Python, this is a mandatory import to be made.
- strftime : The strftime() method in Python returns a string representing the date and time using date, time or datetime object. We can import it from the time module in Python, similar to how we imported the sleep method.
Now, another question that would come to your mind is, what is flush in Python?! So, let us see that too --
flush : Theflush() method in Python clears the internal buffer of the python file. Usually, the files are automatically flushed in Python while closing them, but you may explicitly flush a file before even closing it, by using the flush() method in Python. We will look into a very interesting example using the flush() method ahead in this article!!
Buffer is a place to store something temporarily. It is done when the input and output speeds are different. For example, while watching any video online, the video service downloads the next few minutes of the content to avoid any delays.
So, our code is printing the time and date after every 1 second, depicting a digital clock. However, please note, it will go on continuously because of the while True: condition given into our code, so it is highly recommended to flush out any memory captured by our code every time. It is also recommended to put some break conditions inside your while loop, otherwise it will run infinitely.
sleep() in a multithreaded program
Before getting started with our code examples, let us get a short overview on multithreaded programs and few important terminologies in multithreading.
- Process : A computer program is a collection of multiple instructions. And the process is the execution of those instructions.
- Thread : A thread is basically a subset of the process (also known as light-weight process). A process can have one or more threads.
- Multithreading : Multithreading is a process of executing multiple threads simultaneously.
- Why Multithreading? : The general idea to imply multithreading is to achieve parallelism by dividing a process into multiple threads.
Now, you have learned enough basics to get started with sleep() in a multithreaded program. Till now, the examples we have been seeing were single threaded programs. To work with multithreaded programs in Python, firstly we have to import the thread module in Python. Let us look at a basic example of multithreading in our Python code.
Code:
Output:
Explanation: In the above code, we have created two threads, th1 and th2. They can be run using th1.start() and th2.start() respectively. Please note that, since the two threads th1 and th2 runs parallelly, the order of the output attained might not be fixed. That means, we can get different output sometimes when we run the code.
So, let us see, how can we use sleep() method in a multithreaded code !!
In the case of a multithreaded program, the sleep() function basically suspends the execution of the current thread for a given number of seconds. Please note, it suspends the thread, and not the whole process in multithreaded programs.
Fact: In case of a single-threaded program, the sleep() method suspends the execution of both the thread and process.
Now, let us check out the code to implement multithreading coupled with sleep() in Python.
Code:
Output:
Explanation: In the above code, we again have two threads th1 and th2. Now, please note that this time we have added delays using sleep() into both of our threads. So, this time there will be a particular amount of delay while running both the threads. We have specified the time delay of 0.3 seconds and 0.5 seconds in both the codes respectively. So, any number of times you run the code, you will find them executing in a certain manner, according to the delay provided to them.
Adding a Python sleep() Call With Decorators
Recap of Decorators in Python:
A decorator in Python is a function that takes another function as an argument and extends its behaviour without explicitly modifying it. It is one of the most powerful features of Python. Decorators are usually called before the definition of a function we want to decorate.
In this section, we will learn how can we use the time.sleep() with decorators in Python. Let us look into our code example and then understand it further.
Code:
Output:
Explanation: In the above example, wait() is our decorator, which takes the number of seconds to wait as the input. Inside that, we have our real decorator the decorator function that takes a function and passes it to the wrapper function. Now, the wrapper function takes *args(0 or more number of arguments) as the parameter. Also, the wrapper implies a time.sleep() method for 3 seconds, and then returns the function passed with the argument passed to it.
- For that, firstly we call our wait() function, passing the amount of delay, and store the result in calling_wait_function variable.
- Then, we call our decorator, passing our custom function myFun(), and store the result in the calling_decorator_function variable.
- After that, we finally call our wrapper function. The wrapper function expects *args, which means, 0 or more arguments and store the result in calling_wrapper_function variable.
- And then we get our desired result, after 3 seconds delay.
Now that we have created our wait() decorator, let us use this decorator in some another function. Python provides a much easier way for us to apply the decorators. We can simply use the symbol before the function we would like to decorate. Let us look at the example below.
Code:
Output:
Explanation: In the above code, we have simply used our wait() decorator with the symbol. To look into the wait() decorator's implementation, please refer the code above. As expected, the result will be printed after a delay of 3 seconds.
Adding a Python sleep() Call With Async IO
Recap of Async IO in Python :spiral_note_pad: :
The asyncio is a library to write concurrent code using the async/await syntax in Python. asyncio is used as a foundation for multiple Python asynchronous frameworks that provide high-performance network and web servers, database connection libraries, etc.
Important: The asyncio module provides a framework that*revolves around the event loop. An event loop basically waits for something to happen and then acts on the event.
Let us look at an example where we use Python's sleep method with Async IO.
Code:
Output:
Explanation: The main difference between time.sleep() and asyncio.sleep() is that time.sleep() is blocking, and asyncio.sleep() is non-blocking.
When time.sleep() is called, it will block the entire execution of the script and the script will be put on hold, just frozen, and doing nothing.
Whereas, when we call the await asyncio.sleep(), it will ask the event loop to run something else while our await statement finishes its execution. Hence, you can see in the above code that await asyncio.sleep() is not blocking the execution of the script. Hence, while some part of our code is sleeping , the rest is being executed by the function.
Whereas, if we had used time.sleep() instead of asyncio.sleep(), our code would look something like :
Code:
Output:
Explanation: When time.sleep() is called, it will block the entire execution of the script and the script will be put on hold, just frozen, and doing nothing. Hence, our code is put into hold completely for the specified amount of time, and only when that delay gets over, the next part of our code is executed. That is why, we get all the print statements in sequence to how we wrote them.
Conclusion
In this article, we learned about the time.sleep() method in Python. Let us just recap on what we have seen throughout the article.
- The sleep() function in Python suspends the execution of the current thread for a given number of seconds
- To use the sleep() function in Python, we need to import the time module.
- We can use the time.sleep() function with lists, tuples, decorators, multithreaded programs, acyncio, etc.
- The time.sleep() function is highly useful in cases such as while handling web-APIs, while downloading some files, etc. There are multiple scenarios where we can use it to produce delays in our code.
See Also
Now that you have learned about sleep method in Python, I would encourage you to go forward and pick the below scaler articles to further enhance your knowledge: