Staticmethod in Python
Learn via video course
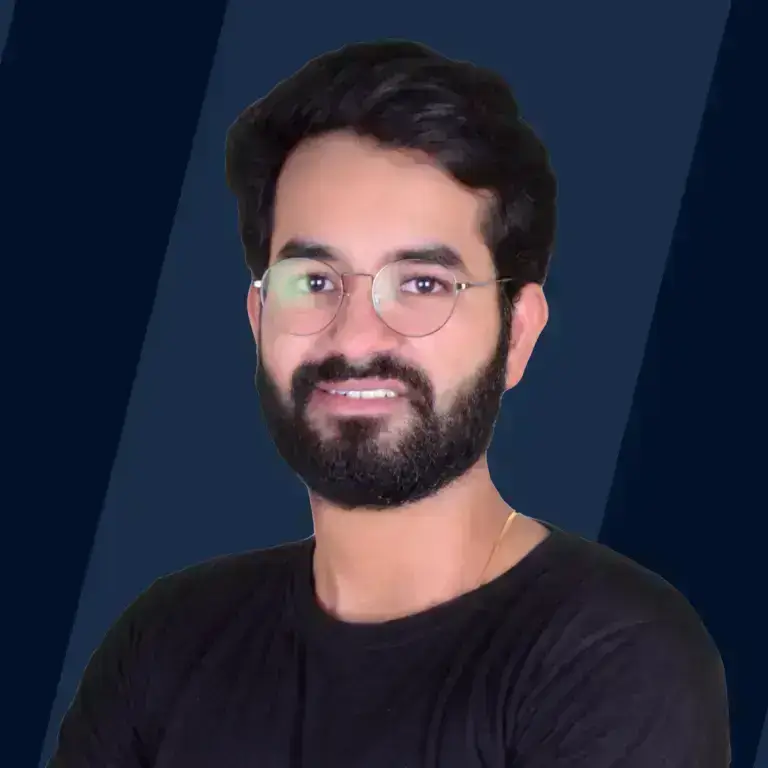
Overview
The static method in Python is similar to class-level methods in python, the difference being that a static method is bound to a class rather than the class objects. This means that a static method can be called without an object for that class and that static method cannot modify the state of an object as they are not bound to it.
Syntax of Staticmethod() in Python
Syntax of @staticmethod is:
Note: staticmethod() is considered an un-pythonic form of creating a static function. Hence, in newer versions of Python, you can use the @staticmethod decorator.
Parameters of Staticmethod() in Python
The staticmethod() method takes a single parameter:
function - function that needs to be converted into a static method
Return Values of Staticmethod() in Python
The staticmethod() returns a static method for a function passed as the parameter.
Exceptions of Staticmethod() in Python
The static method cannot access the class attributes or instance attributes. It will raise an error if try to do so.
Example of Staticmethod() in Python
The following example demonstrates the working of staticmethod() in python:
Output:-
What is a Static Method in Python?
Static methods are methods that are bound to a class rather than its object. They do not require a class instance creation. So, they are not dependent on the state of the object.
Static methods in Python are similar to python class-level methods, the difference being that a static method is bound to a class rather than the objects for that class. This means that a static method can be called without an object for that class and also that static methods cannot modify the state of an object as they are not bound to it.
The main difference between a static method and a class method is:
- Static method knows nothing about the class and just deals with the parameters.
- The class method works with the class since its parameter is always the class itself.
They can be called both by the class and its object.
Python Static Methods
-
Using staticmethod()
The code snippet below demonstrates how to use staticmethod() in python:
Output
In the above program, we converted the add function into a static method and called the Test.add function without creating an object of the class. This approach is controlled as at each place, it is possible to create a static method out of a class method as well.
-
Using @staticmethod
The code snippet below shows a more subtle way of creating a static method:
Output
The above program uses @staticmethod which is a built-in decorator that defines a static method in the class in Python. A static method doesn't receive any reference argument whether it is called by an instance of a class or by the class itself. The add function cannot have self or cls parameter.
When Do You Use the Static Method in Python?
- Grouping utility function to a class Static methods have a limited use case because, like class methods or any other methods within a class, they cannot access the properties of the class itself. However, when you need a utility function that doesn't access any properties of a class but makes sense that it belongs to the class, we use static functions.
- Having a single implementation Static methods are used when we don't want sub-classes of a class to change/override a specific implementation of a method.
- Consume Less memory Instance methods are object too, having a static method avoids the cost of creating multiple copies of instance methods in a class.
More Examples
-
How to Call Static Method from Another Method?
Let us see how to call a static method from another static method of the same class with the help of an example.
Output
-
Calling Static Method from Instance Method
In the above program, have created a static method get_tasks() that accepts the to-do list name as a parameter and returns all the tasks to be completed. We called the static method from instance method start().
Conclusion
- The staticmethod() built-in function returns a static method for a given function.
- A static method can be called without an object for that class and that static method cannot modify the state of an object as they are not bound to it.
- Static methods have limited use, because they don't have access to the attributes of an instance of a class (like a regular method does), and they don't have access to the attributes of the class itself (like a class method does).