String to JSON Python
Learn via video course
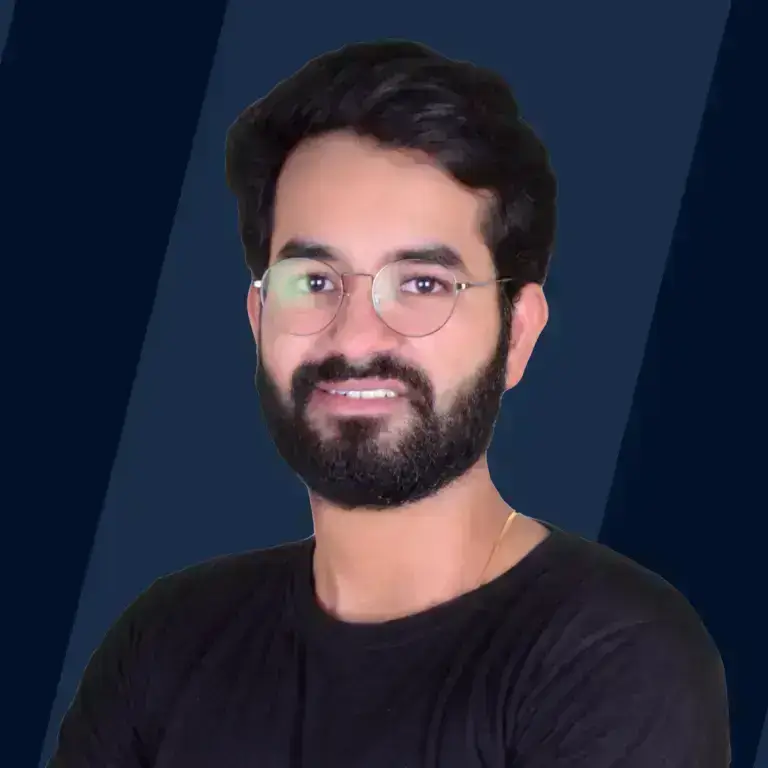
Overview
JSON (JavaScript Object Notation) is a data format often used for sending and receiving data between servers and applications. Python provides an in-built package called json to handle data in JSON format. The two most important functions provided by this package are json.dumps() and json.loads().
Scope
In this article, we will discuss:
- What is JSON and where it is used.
- How to convert JSON data into Python dictionaries and vice versa using the native json package of the Python language.
- We will also see another method called eval to convert a JSON string to a Python dictionary.
Introduction to JSON
Most of the applications today are divided into two domains:
- Backend
- Frontend
Now, these two parts of an application need to communicate in some way. Therefore, we require a way to send data back and forth.
JSON (JavaScript Object Notation) is a popular and standardized format used for transmitting and receiving data between a server and an application or between two applications.
The following diagram shows the case of an application communicating with the server using data represented in JSON format:
A JSON object is very similar to a Python object. It also contains key-value pairs
For instance, the following is a JSON object:
This JSON object contains details about a person named "Thomas Shelby". It has the age, organisation, and a list of partner associated with this person.
You can notice similar types of values: string, numbers, lists, etc.
The following table shows the type conversion between Python and JSON:
Python | JSON |
---|---|
dict | object |
list, tuple | array |
str | string |
int, long, float | number |
True | true |
False | false |
None | null |
Note that in a Python dictionary you can use a single or double quote to define the keys of a dictionary but in a JSON object you must use double quotes only.
Takeaway
- JSON is a standardized format used for transmitting and receiving data between a server and an application.
Converting a Python Dictionary to a JSON Object represented as a String
Python comes with a built-in package called json that we can import to use its function for handling JSON data.
The process of converting a Python dictionary into a JSON object is called serialization.
The term "serialization" refers to the transformation of data into a series of bytes to be stored on disk or transmitted across a network.
We will use the json.dumps function to convert a dictionary into a JSON object represented inside a string.
Syntax:
This function returns a JSON formatted string.
Let's see an example.
Code:
Output:
Explanation:
We created a Python dictionary with details of a person and passed it to the json.dumps function, this function will return a JSON version of the dictionary. We stored the returned JSON object in a variable and printed it.
Takeaway:
- We can convert a Python dictionary into a JSON object using the dumps function from the json package.
Converting a JSON Object represented as a String to a Dictionary in Python
Now, let's see how we can convert a JSON object available in a string to a Dictionary in Python.
The process of converting a JSON object into a Python dictionary is called deserialization.
Method 1: String Object to Dict Object Using json.loads
The json package also provides a function called json.loads for decoding a JSON object present in a string into a Python Dictionary.
Syntax:
Let's see an example to get a clear understanding of the same.
Code:
Output:
Explanation:
We have a JSON object stored inside a string, we passed this string to the json.loads function. This function returned the equivalent Python dictionary. Then, we printed the dictionary.
Tip You can use the pprint package to pretty-print the dictionary in the previous example to make it more readable:
Code:
Output:
Method 2: str Object to dict Object using eval()
We can also convert a JSON string into a Python dictionary using the in-built eval function.
Syntax:
where expression is any valid Python code.
Let's see the example.
Code:
Output:
Explanation:
eval() allows us to evaluate arbitrary Python expressions from a string-based input. Here, we used it to parse a dictionary inside a string to an actual dictionary. Then, we printed the returned dictionary.
Note
The function eval() is a lot versatile than the previously seen json.loads. It can be used to run any Python code and is not limited to converting a JSON string to a dictionary.
For instance, you can use eval() to perform calculations as follows:
Output:
Takeaway:
- We can convert a JSON object into a Python dictionary using the loads function from the json package or using the eval function.
Conclusion
- JSON is a popular and standardized format used for transmitting and receiving data between a server and an application.
- A JSON object is similar to a Python dictionary containing key-value pairs.
- The process of converting a Python dictionary into a JSON object is called serialization.
- We can convert a Python dictionary into a JSON object using the dumps function from the json package.
- The process of converting a JSON object into a Python dictionary is called deserialization.
- We can convert a JSON object into a Python dictionary using the loads function from the json package or using the eval function.