Difference Between Throw and Throws in Java
Learn via video course
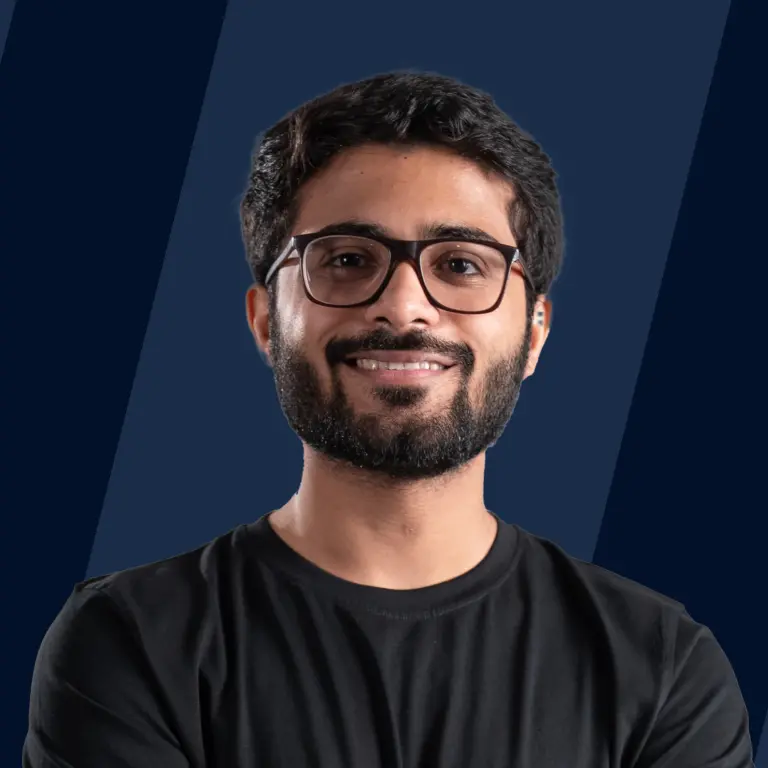
Overview
Every program can have run-time and/or compile-time errors. Mostly, compile time errors are solved by the developers while developing or testing the program but what about run-time errors ? Run time errors are based on the inputs which we provide to our program and it is quite normal for a program to have, in an actual environment, inputs which it can not handle.
For these types of situations, Exception handling comes to the story. As a responsible developer it is our duty to minimize these exceptions as possible as we can and handle all the possible exceptions that we can think of. In order to achieve this goal, Java has an exception handling mechanism which includes keywords such as try, catch, throw, throws etc.
throw and throw keywords are used for exception handling, in which the former is used for throwing exceptions explicitly while the latter is used as a signature for methods.
Scope
- In this article, we will learn about the functions and uses of the throw and throws keyword.
- We will study the differences between these keywords.
- We will try to understand the differences between checked and unchecked exceptions in java.
- We will also learn about the propagation of exceptions in java.
Introduction
This article explores the differences between two seemingly similar keywords in java. The Throw keyword and the Throws keyword. Both are used as part of the exception handling mechanism in java.
For example, you are asked to write a program that performs division on two numbers and returns the output. In the logic of the program, there is a case of division by 0 which is an invalid operation.
So there is a risk that while we ask the user for input for the two numbers to perform division on, the user might accidentally give the denominator as 0, thereby abruptly ending the program execution with an error.
Exception Handling provides a mechanism to gracefully handle such issues that might arise in a program like cleaning up any allocated memory and showing the exact error message to the user that caused this exception etc. The Throw and Throws in Java are two keywords that can be used to do exception handling.
Throw: The Throw keyword in java is used to explicitly throw an exception inside a method or a block of code in a java program.
Throws: The Throws keyword in java is used at the method signature to declare all the exceptions that might be thrown by the method during its execution.
Both keywords are used to handle exceptions that occur in a method or a block of code, both have their functionality and their usage. Let us look at the differences between them and their usage with examples.
Difference Between Throw and Throws in Java
A. Syntax:
Throw: The syntax of the throw keyword is, keyword throw followed by the instance of the exception that needs to be raised. Below is the syntax:
Example:
Throws: The syntax of the throws keyword is, keyword throws followed by class names of the exceptions.
Example:
B. Declaration
Throw: The throw keyword is used inside a method or a block of code.
Throws: The throws keyword is used with the method signature.
C. Point of usage
Throw: The Throw keyword is used inside a method or a block of code. It is used when there is a requirement to throw an exception explicitly.
Example Java Code Snippet that illustrates the usage of Throw:
Explanation: Inside the method test_method() few statements require an exception to be thrown, so the “throw” keyword is used to throw an ArithmeticException();
Throws: The Throws keyword is used at the method/constructor signature. It is used for such a method where an exception might arise during the execution.
Example Java Code Snippet that illustrates the usage of Throws:
Explanation: Inside the test_method() few statements might cause an ArithmeticException. For example, the statement can be a division of two numbers. The division of two numbers statement is prone to division by zero which is invalid, so in the function signature “throws” with ArithmeticException is added.
D. Types of Exceptions
Exceptions in Java can be classified into two types
1. Checked Exception:
This is an exception that occurs at the time of compilation and is also called Compile-time Exceptions. These exceptions must be handled using some exception handling mechanism in the program otherwise the program gives compile-time errors.
For example, while reading a text file in java and using FileReader, the FileNotFoundException(when the file is not present in the given file path) needs to be handled using some exception handling mechanism otherwise a compile-time exception is thrown. ClassNotFoundException and SQLException are some other checked exceptions.
2. Unchecked Exception:
These occur at the time of execution. These are also called Runtime Exceptions. Runtime exceptions are ignored at the time of compilation. For example, invalid operations like division by zero, accessing an array with an index bigger than its size, etc are all actions that are all runtime/unchecked exceptions.
Note The throw keyword can be used to throw both checked and unchecked exceptions.
Program: Below is a sample java program that we can use to manually throw a NullPointerException which is an unchecked exception
Output:
Explanation:
- We created a String variable temp and assigned it null.
- NullPointerException as we know is an Unchecked exception so it won’t give any compile-time errors if not handled.
- But here we are using the throw keyword to manually throw the exception if the variable temp is null.
Note: The handling of checked exception using throw keyword is used when propagating an exception from one function to another. However only the throw keyword cannot directly propagate a checked exception, It is done along with using the throws keyword. Please refer to section f of the article for the example and explanation for this point.
Note Using the throws keyword we only need to declare and handle checked exceptions.
Program: Below is a sample java program that reads a file from a given path.This illustrates the use of throws to handle the checked exception(FileNotFoundException):
Output:
Explanation:
FileNotFoundException is a checked exception that needs to be handled at the compile time. In the above program, we used the “throws FileNotFoundException” clause added to the main method. We used the File class and FileInputStream class to create a file1 object with the given path. And if the file is not present in the given path then the FileNotFoundException will be thrown.
E. Number of Exception that can be handled at a time
Below is how the throw and throws work in terms of the number of exceptions they can handle at a time.
Throw: Only one exception can be thrown using the throw keyword. We cannot throw multiple exceptions using the throw keyword.
Throws: Throws keyword can handle multiple exceptions. Multiple exceptions can be declared at the method signature separated by the comma operator.
F. Propagation of Exceptions
Whenever an exception occurs, first it is thrown from the top of the stack. If it is not caught there, it goes down in the call stack and checks for the previous method. If it is not caught there as well, it again goes down in the call stack and checks for the previous method until it is caught somewhere or reaches the very bottom of the stack. This flow is known as Exception Propagation.
From the above example we can understand the concept of Exception Propagation. We are having ArithmeticException in the firstMethod() but we are catching that exception in our main() method as system looks for the appropriate catch block in the secondMethod() and thirdMethod(), respectively, but do not find it there.
Below is how throw and throws propagate the types of exceptions:
- The throw keyword propagates unchecked exceptions only. Checked exceptions cannot be propagated in the call stack using throw directly.
- The throws keyword allows both checked and unchecked exceptions to be propagated in the call stack.
Note: The throw keyword can be used to propagate a checked exception provided that exception is declared using throws at the signature of the method which has a statement that caused that. The below program illustrated this point and shows how throw and throws are used for exception propagation.
Program:
Output:
Explanation:
- The main method calls another method fileCheck() inside a try-catch block.
- Inside the file check, we tried to read a file from the given path, which is the code that raises a checked exception, which is caught using the try-catch block.
- However, we have used the throw keyword to propagate the FileNotFoundException to the parent/caller method which is the main method.
- So, we declare the ‘throws IOException’ (FileNotFoundException extends IOException class) in the fileCheck() function signature, because throw cannot directly propagate a checked exception without the throws declared at that function signature.
- The exception is then propagated and caught at the try-catch block written inside the main method where it is handled.
Difference Between Throw and Throws in Java Explained
Topic | throw keyword | throws keyword |
---|---|---|
Definition | Throw keyword in Java is mainly used for throwing exceptions explicitly at the time of program execution. | Throws keyword in Java is mainly used for declaring known possible exceptions that can occur at the time of program execution. |
Usage | It is used within the method definition. | It is used with method declaration. |
Syntax | It is followed by throwable objects. | It is followed by the class name of Exceptions which is going to be thrown. |
Implementation | throw throwableObject;-> It allows throwing only one exception at a time. | acess_modifier return_type method_name () throws exception_type1, exception_type2, …, exception_typeN {//Java_Code} ->We can declare multiple exceptions separated by commas using the throws keyword. |
Exception Handling | If we are using throw keyword, then we must write appropriate catch blocks to handle that exception. | If we are using throws keyword, then the calling method will handle the exception. |
Occurrence of Exception | Whenever system encounters a throw keyword, it throws the exception explicitly. | Whenever system encounters throws keyword, it throws exception only when it actually occurs |
Examples of Java throw keyword
Let us dive into the practical usage of throw keyword in Java language:
Here, we can understand how the keyword is actually worked and understand how the flow works through examples.
Example 1: Throwing custom exception while trying to check valid driving age
In the above example, initially, declared UnableToDriveException which is the custom exception class and it just extending Exception class calling the parent constructor and returning string usingsuper().
In CustomTestException class, we take age as a input and checking with minimum age for driving and if fails, will throw UnableToDriveException using throw keyword.
Example 2: Throwing "divide by 0" Arithmetic exception
Output:
Example of Java Throws Keyword
Below we have listed some common examples for which Java throw keyword is used in order to handle exceptions properly.
Example 1: Java throws Keyword
Above code will take two integers from the user and print their division. During division if the user inputs a second integer as 0 then an exception message will be thrown by the system as division by zero is not possible.
If the user inputs a second integer apart from 0 then code will run normally and exceptions will not occur and the system will print the result of the division on the screen.
Example 2: Throwing multiple exceptions
Above code will give the division of the numbers provided by the users. It is taking three values from the user,
- Dividend as a first number
- Divisor as a second number
- Length of the array as a third number
Case 1: if the user gives appropriate numbers for division and length of the array more than 3 as input parameters, the program will run correctly and print the result of division on the output screen.
Case 2: if the user gives the second number as 0 and/or length of the array less than 3 then our custom method will throw exceptions and will be caught by the main() method. As all the exceptions in Java extends Throwable class e.g. ArithmeticException, ArrayIndexOutOfBoundsException will be caught by the catch block.
Example 3: Handling Multiple exceptions using throws
Above code will give the division of the numbers provided by the users. It is taking three values from the user,
- Dividend as a first number
- Divisor as a second number
- Length of the array as a third number
Case 1: if the user gives appropriate numbers for division and length of the array more than 3 as input parameters, the program will run correctly and print the result of division on the output screen.
Case 2: if the user gives the second number as 0 and length of the array more than 3 as input parameters, our custom method - ThrowsExample will throw Arithmetic Exception (because division by zero is not possible mathematically) and will be caught by the main() method.
Case 3: if the user gives appropriate numbers for division but length of the array less than 3 as input parameters, our program will be able to do division correctly but as we are storing our result of division in the 3rd index of the array, our custom method - ThrowsExample will throw ArrayIndexOutOfBounds Exception (because our array has not enough space in order to save our division result) and will be caught by the main() method.
Conclusion
We have reached the end of the Article.
-
In this article, we explored the differences between the throw and throws keyword in java.
-
We also learned about checked and unchecked exceptions in java.
-
We studied the propagation of exceptions in java.
-
Below are some example java programs you can try to implement and check to see how they can be handled using throw and throws.
- Java program to handle checked exception ClassNotFoundException using throws keyword.
- Java program to handle unchecked exceptions ArrayIndexOutOfBoundsException, NumberFormatException using throw keyword.
- Java program to propagate an Unchecked Exception like NullPointerException using throw keyword.