Data Structures in Python
Learn via video course
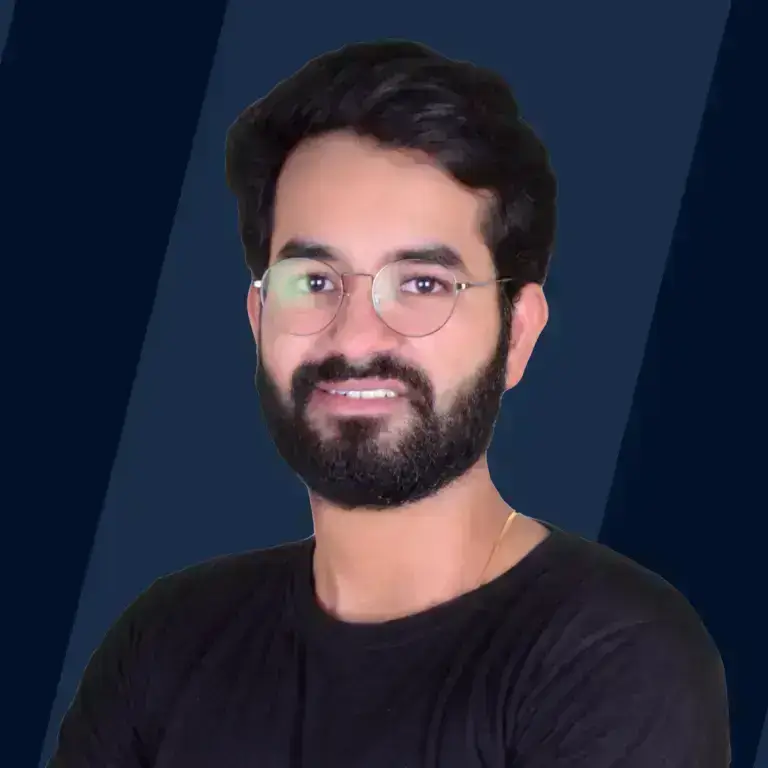
Data Structures in Python
Overview
The data structures in python are a meaningful way of arranging, and storing the data in the computer for efficient utilization and processing depending upon the situation.
Scope
This article cover the below topics:
- Data structures in python and Different categories of data structure in python.
- Built-in data structures and User-defined data structures.
- Sets, lists, tuples, dictionaries, stack, queues, trees, graphs, hashmaps, etc. data structures in python.
What is a Data Structure?
Before learning about data structures in python, we should first know what is data? Data is a piece of information translated into a form that is efficient to translate and process.
The data structures in python are nothing but a meaningful way of arranging, and storing the data in the computer for efficient utilization and processing depending upon the situation.
The data structure is one of the most fundamental topics of any programming language. The data structures in python language can be broadly divided into two categories.
We have four built-in (general) data structures in python namely - lists, dictionaries, tuples, and sets. Apart from these, we have some user-defined data structures in python like stacks, queues, trees, linked lists, graphs, hashmaps. Let us broadly discuss data structures in python, the types of data structures in python in detail.
Refer to the detailed data structure article for reference.
Types of Data Structures in Python
As we have discussed, the data structures in python can be broadly divided into two categories, the first one being the built-in data structures in python, and the second one being user-defined data structures in python. The data structures in Python are:
1. Built-in Data Structures
-
List in Python - The Lists is a built-in data structures in python that stores the data in the sequential form. Lists can be treated as dynamic arrays. List data structures in python can contain duplicate elements as each element is identified by a unique number/ position known as an index. The list in python provides a wide variety of methods and functions. We can put heterogeneous type values inside the lists. The most common associated method of lists are list(), append(), insert(), extend(), sort(), reverse(), remove(), pop(), clear() etc.
-
Dictionary in Python - The dictionary is also a built-in data structures in python that stores data in the form of key-value pair. The dictionary data structures in python have an attribute called the “key”. The key is the attribute that helps us locate the data or value in the memory. The dictionary in python provides a wide variety of methods and functions. The keys are always unique within a dictionary. The values of the Python dictionary may or may not be unique. We can put heterogeneous type values inside the dictionaries. The most common associated method of dictionaries are get(), keys(), values(), items(), update(), pop(), clear() etc.
-
Tuple in Python - The tuple is also a built-in data structures in python which are somewhat similar to lists data structures in python. A tuple is an immutable object that stores the elements in an ordered manner. We cannot change the elements of the tuple or the tuple itself, once it's assigned whereas we can change the elements of the list as they are mutable. The tuple in python provides a wide variety of methods and functions. We can put heterogeneous type values inside the tuples. The most common associated method of tuples are tuple(), count(), index() etc.
-
Sets in Python - The set is also a built in data structures in python. A set is a collection of unordered values or items. The set in python has similar properties to the set in mathematics. The set in python provides a wide variety of methods and functions. The set() function is used to create a set in python. We can put heterogeneous type values inside the sets. The most common associated method of sets are set(), add(), update(), remove(), pop(), discard(), clear(), intersection(), union(), set_difference() etc.
2. User-Defined Data Structures
-
Stack in Data Structure - Stack is not a built i- data structures in python. Stack is a user-defined data structures in python that are used to store data in the Last In First Out (LIFO) manner. In stacks, the insertion and deletion are done at one end, where the end is generally called the top. Since the last element inserted is served and removed first, the stack data structures in python are also known as the Last Come First Served data structure. The most common associated method of stacks are push(), pop(), peek(), isEmpty(), isFull(), size() etc.
-
Queue in Python - Queue is also not a built-in data structures in python. The queue is a user-defined data structure in python that is used to store data in the First In First Out (FIFO) manner. In queues, the insertion and deletion are done at different ends, where the insertion is done at the rear end and the deletion is done at the front end. So, the data which is inserted first will be removed from the queue first. Since the first element inserted is served and removed first, the queue data structures in python are also known as the First Come First Served data structure. The most common associated method of queues are enqueue(), dequeue(), front(), rear(), empty() etc.
-
Tree Data Structure - Tree is also not a built-in data structures in python. A tress can be defined as a collection of objects or elements or entities known as nodes. These nodes are linked together to represent a hierarchy. The top node of the hierarchy is known as the root node. In trees, each node contains some data and also contains references to its child node(s). A root node can never have a parent node. the nodes that do not have any child nodes are termed leaf nodes. A tress can be of various types such as a binary tree, binary search tree, AVL tree, Red-Black tree, etc.
-
Linked List - Linked List is also not a built-in data structures in python. A linked list is a linear structure of data consisting of nodes. Each node of a linked list contains some data and also contains references (pointer to address) to the next node. The first node of a linked list is called the Head node, which helps us to access the entire linked list. The last node of the linked list is called the Tail node, which resembles or marks the end of the linked list. Since the last node marks the end of the linked list, it points to a NULL value or None! A linked list can be of various types such as singly linked list, doubly linked list, circular linked list, doubly circular linked list, etc.
-
Graph Data Structure - Graph is also not a built-in data structures in python. A graph is a non-linear structure of data consisting of nodes that are connected to other nodes of the graph. The node of the graph is also known as vertex (V). A node can represent anything such as any location, port, houses, buildings, landmarks, etc. They are anything that we can represent to be connected to other similar things. Edges (E) are used to connect the vertices. The edges represent a relationship between various nodes of a graph. A graph can be of various types such as a directed graph, undirected graph, directed acyclic graph, directed cyclic graph, etc.
-
HashMaps - Hashmap is also not a built-in data structures in python. A hashmap is also known as a hash table. A hashmap is one of the data structures in python that maps keys to its value pairs. It is somewhat similar to a dictionary since dictionaries also store key-value pairs but hashmaps use a function to compute the index value that stores or holds the elements to be searched. This function or hashing() helps to retrieve mapped elements in a faster manner than the normal sequential or linear search. Simply we can say that hash tables store key-value pairs and the key is generated using a hash function. In python, we use the built-in dictionary data structures to implement hash maps. The elements of a hashmap are not ordered and can be changed.
Conclusion
- The data structures in python are a meaningful way of arranging, and storing the data in the computer for efficient utilization and processing depending upon the situation.
- The data structures in python language can be broadly divided into two categories.
- We have four built-in (general) data structures in python namely - lists, dictionaries, tuples, and sets.
- Some user-defined data structures in python are stacks, queues, trees, linked lists, graphs, hashmaps.
- List is a built-in data structure in python that stores the data in sequential form.
- The dictionary is also a built-in data structures in python that stores data in the form of key-value pairs.